Follow along with the Exploring JavaScript Array Methods series!
- Exploring Array ForEach
- Exploring Array Map
- Exploring Array Filter
- Exploring Array Reduce
- Exploring Array Some
- Exploring Array Every
- Exploring Array Find (you’re here)
What is Array Find?
Array Find is a method that exists on the Array.prototype
that was more recently introduced in ECMAScript 2015 (ES6) and is supported in all modern browsers.
Array Find searches your array and returns you the first matching element, or undefined
. Find’s return value is dynamic and could be of any JavaScript type that exists in your array, a string, number, object etc.
Think of Array Find as: “I want to find a particular element in my array”
In ways, Array Find is similar to Array Filter, however returns just the first result, whereas Filter would return you as many results that satisfy the test!
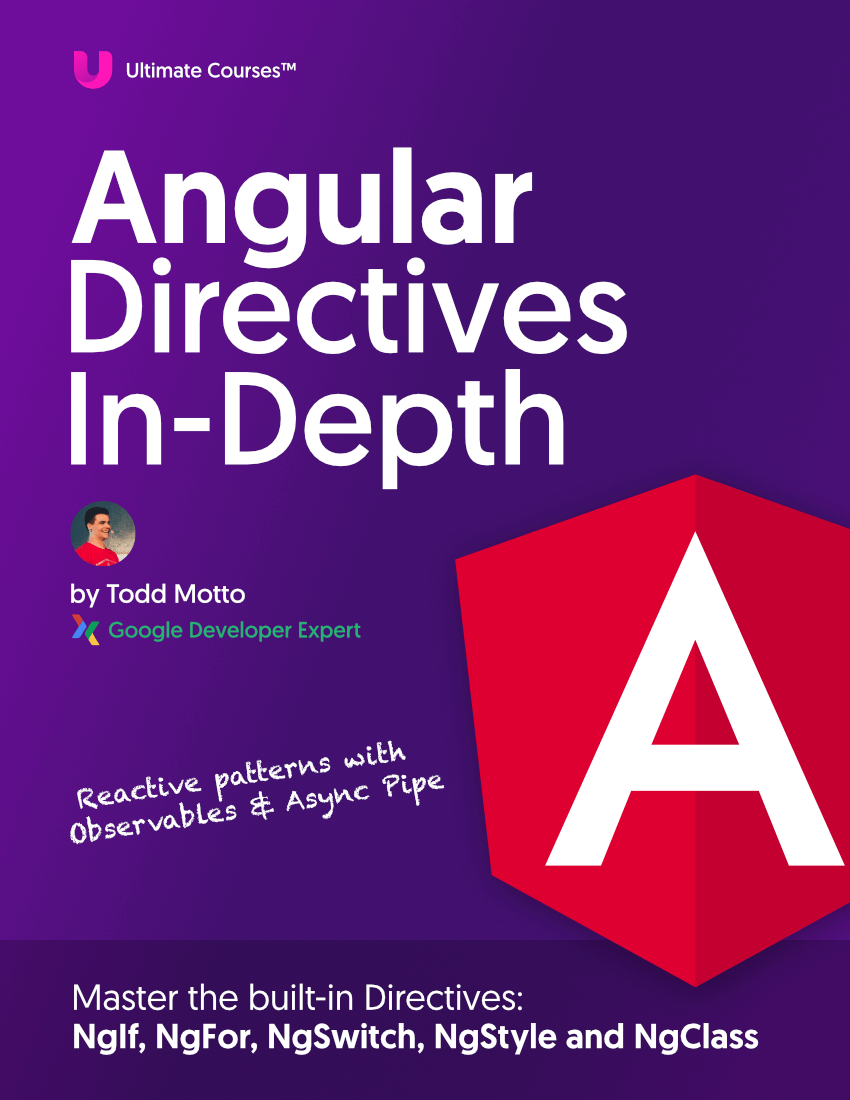
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Here’s the syntax for Array Find:
const foo = array.find((value, index, array) => {...}, thisArg);
The value const foo
will contain any element from your array, and therefore it could be of any value type.
Array Find syntax deconstructed:
- Find’s first argument is a callback function that exposes these parameters:
value
(the current element)index
(the element’s index - not commonly used with Find)array
(the array we are looping - rarely used)- Inside the body of the function we need to
return
an expression which will evaluate to a Boolean (true
orfalse
), this will then tell Find what to return after completing the loop
- Find’s second argument
thisArg
allows the this context to be changed
See the ECMAScript Array Find specification!
In its simplest form, here is how Find behaves:
const found = ['a', 'b', 'c', 'd'].find(x => x === 'a');
// 'a'
console.log(found);
Find will return us a “shallow copy” of 'a'
- which is always the case with any value in our array. We are always passed a copy rather than a direct reference - which helps us mitigate potential bugs.
It will also return undefined
if, for example with the value 'e'
, the result does not exist in the aray:
const notFound = ['a', 'b', 'c', 'd'].find(x => x === 'e');
// undefined
console.log(notFound);
As Find returns any value type, it has very flexible use cases! You could return Booleans, Strings, Objects, Arrays to any degree - but a common use case could be finding an object inside an array by supplying an ID to lookup the object with. We could, for example, then use the return value to perhaps update that particular element or send it to a server.
As soon as Find successfully ‘finds’ a first element match, it will return it to you - so keep this in mind when dealing with duplicate array items, as you will only get one result back from Find. Find will also loop in ascending order, so there should be no surprises.
Using Array Find
Here’s our data structure that we’ll be using Array Find with:
const items = [
{ id: '🍔', name: 'Super Burger', price: 399 },
{ id: '🍟', name: 'Jumbo Fries', price: 199 },
{ id: '🥤', name: 'Big Slurp', price: 299 }
];
We could find any item we like, via any of the properties available.
Let’s use Find to find an item based on it’s id
property:
const found = items.find((item) => item.id === '🍟');
// { id: '🍟', name: 'Jumbo Fries', price: 199 }
console.log(found);
As found
could also contain undefined
, it’s best practice to safety check the variable in some way:
const found = items.find((item) => item.id === '🍟');
if (found) {
// Jumbo Fries 1.99
console.log(`${found.name} ${(found.price / 100).toFixed(2)}`);
}
Nicely done. If the item is available, let’s do something with the data!
Notice how simple Find is, we’re simply returning item.id === '🍟'
and we immediately get it back once it matches.
Give the live Array Find demo a try:
Bonus: Find-ing without Find
Let’s check out a for...in
loop example that mimics the behaviour of Array Find:
// `undefined` by default
let found;
for (let i = 0; i < items.length; i++) {
const item = items[i];
if (item.id === '🍟') {
found = item;
break;
}
}
First we declare let found
and do not assign a value. Why? Because by default, it’s undefined
- you can explicitly assign it if you like, though.
Inside the loop, we then find the item and assign it to the found
variable, and break the loop - giving us a nice imperative “find” solution.
Summary
You’ve now learned how to use Array Find to grab any particular element you want in your array, in any way you want to find it.
Array Find is a nice and compact way that we can declaratively search through an array and get a copy of the first matched element.
Remember as well, Find is similar to Filter! Filter just gives you all results if they match, rather than the first result only.
If you are serious about your JavaScript skills, your next step is to take a look at my JavaScript courses, they will teach you the full language, the DOM, the advanced stuff and much more!
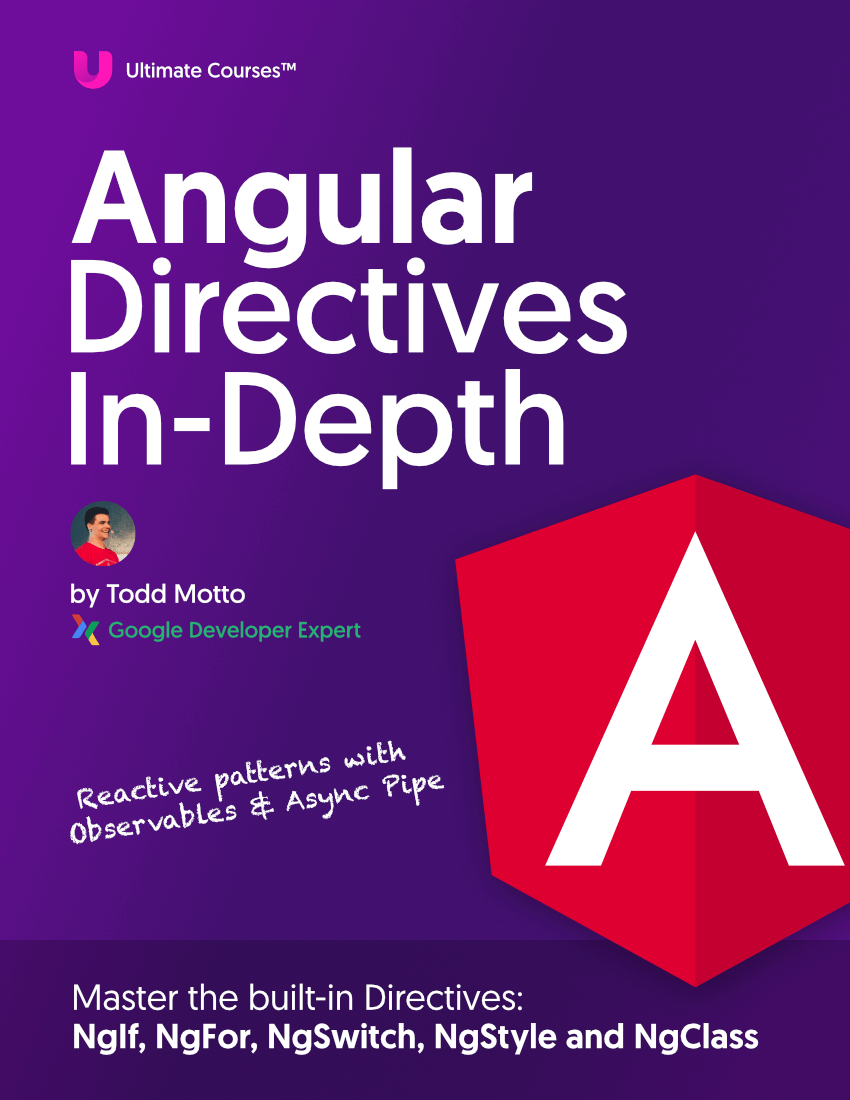
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Further tips and tricks:
- Don’t forget to
return
inside your callback, or your values will beundefined
and evaluate tofalse
- avoid undetected bugs! - You can access the array you’re looping in the third argument of the callback
- You can change the
this
context via a second argument to.find(callback, thisArg)
so that any references tothis
inside your callback point to your object - You can use arrow functions with Find but remember that
this
will be incorrect if you also supply athisArg
due to arrow functions not having athis
context - Using Find will skip empty array slots as if it were a falsy value
- You shouldn’t need to in this day and age of evergreen browsers, but use a polyfill for older browsers if necessary
Thanks for reading, happy Finding!