We will start by understanding what JavaScript is, where it came from, why it’s so wildly popular and begin diving into the components that make up the language - and where we are today.
If you’d like to watch the video version of this article, please watch below! It’s part of my JavaScript Basics course which is a comprehensive guide for beginners and advanced developers.
Table of contents
This introduction guide to JavaScript will answer many questions you have, or will have, such as “What is JavaScript?” and “What are interpreted and compiled languages?”.
I’ll also show how the language fits together and the different styles and paradigms of JavaScript you can write. We’ll also get a brief history lesson on where JavaScript came from and where it is today. We’ll then explore how to write the most modern style of JavaScript we can and how development tools can get us there! This also includes how browsers execute your JavaScript code, there’s lots of great stuff coming up.
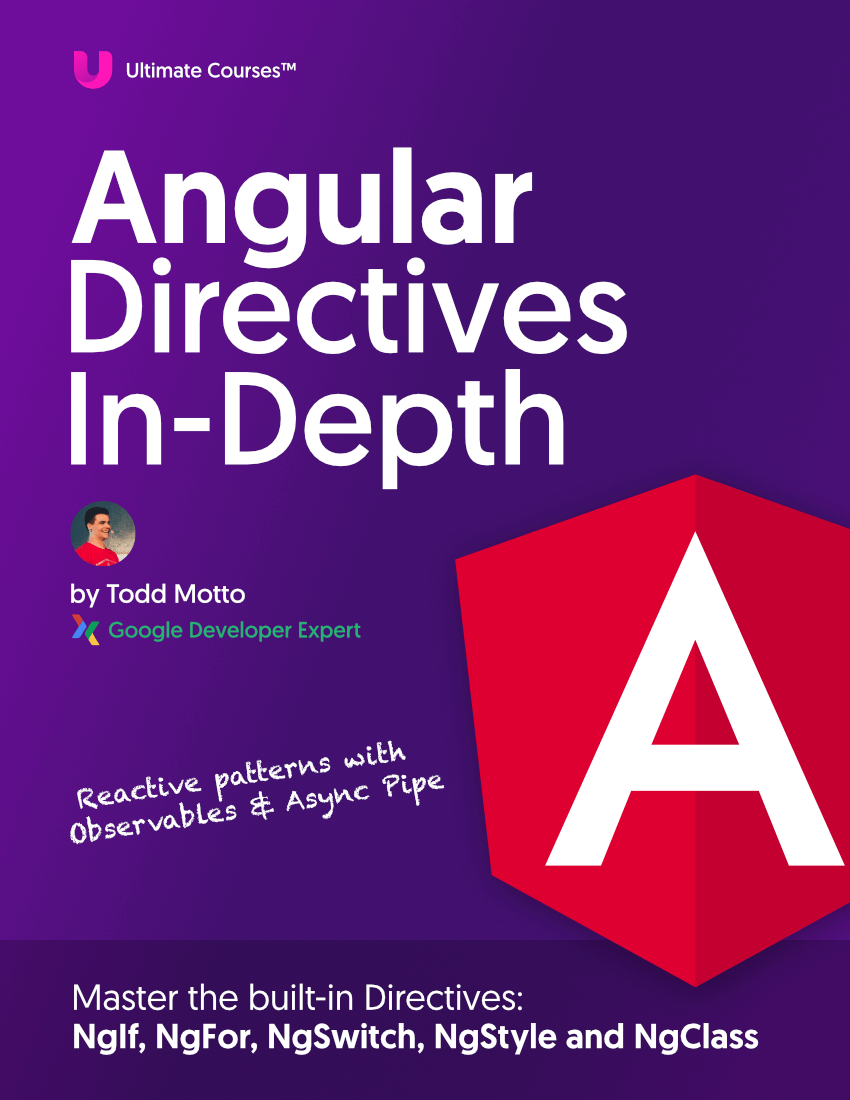
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
So, where do we begin? It makes sense to define the characteristics of JavaScript so we can understand how to work with it.
JavaScript is a high level and interpretive programming language.
JavaScript has types, operators, standard built-in objects and methods.
JavaScript - Interpreted or Compiled?
JavaScript is considered to be an interpreted language because our JavaScript code doesn’t need to be compiled to be executed - unlike some languages such as C, Java, TypeScript and many others.
The key difference in an interpreted language, such as JavaScript, is that there does not need to be a compiler step which translates our JavaScript source into machine code.
Interpreted languages are translated upon execution and tend to be human readable. One downside to interpreted languages is that we can end up with runtime errors.
There are also many upsides for us to consider with compiled languages as we have a slightly different approach. Code that needs a compiler is transformed from source code to native code before the program is actually executed. This has benefits as well such as upfront compile time errors - and also performance.
JavaScript - A Multi-paradigm Language
JavaScript is known as a multi-paradigm language because of its support for multiple programming styles.
If you’re coming from an existing programming background, you’ll likely have a few styles of your own that you could apply to JavaScript. We’ll be learning more about design patterns in JavaScript as we continue, but it’s good to know that JavaScript supports many styles of programming styles such as imperative, procedural, object-oriented (which is supported by prototypal inheritance) - we also have functional programming in JavaScript.
They are simply patterns and methodologies we can apply to our code to make our programming lives easier, learning the fundamentals correctly will allow you to adopt new patterns and techniques the right way, and far quicker. Proper understanding trumps all.
History of JavaScript
The JavaScript language has seen huge improvements and has gained solid momentum in recent years - soaring in popularity to become one of the world’s most popular (if not THE most popular programming language).
JavaScript’s original intention, compared to how it’s evolved and how we use it today, was to allow web pages to become a little more interactive, things such as hovering over an image produced an effect, or a different image. Clicking could create pop-ups and interaction components.
JavaScript was designed to work with HTML and CSS, communicating with something that we call the Document Object Model (DOM). You’re here today to learn about modern JavaScript, but for a moment let’s consider the past and where JavaScript came from.
In a brief history lesson - our story begins in 1995 where a new language was being developed under the code name of “Mocha”. This language was to be named JavaScript someday.
Mocha was initially designed to be interpreted in a web browser. It was then decided to rename Mocha to “LiveScript”, where it shipped in a now ancient browser known as Netscape Navigator. LiveScript was later renamed to JavaScript - which led to much confusion as Java was (and still is) a very popular programming language as well. The two however are not related despite what some may say or think!
Fast forward to 1997 and browser vendors were hard at work all competing to push JavaScript forward.
At some point it was decided that standardising these developments of JavaScript was actually rather a good idea. This led to the creation of something we now call ECMAScript, or ES for short. You may already be familiar with terms such as “ES5” or “ES6”.
ECMAScript is in fact a specification that JavaScript simply conforms to and implements. It’s not a language of its own.
The long story short is we now have a brilliant standards body and a specification for JavaScript to follow. Consider ECMAScript as a set of guidelines that different browser vendors should (very ideally) follow to implement new language constructs and features.
We want browser vendors to all follow the same path as once upon a time the web and JavaScript as an environment to work with were very buggy and a tough landscape to develop in. We want consistency and predictability across all the browsers and environments!
Several years later, in 2009, ECMAScript version 5 was published and lots of fantastic new additions to the JavaScript language were introduced. ECMAScript 5 is now fully supported in all modern browsers.
ECMAScript has continued to advance the language on a yearly basis, and in 2015 ECMAScript version 6 was released - which we now know as and shorten to ES6. Its official name however is ECMAScript 2015, and ES2015 for short. This brought the biggest change to the language since its inception, and 2016 saw the arrival of ECMAScript 2016 - giving us a handful of new features in the language.
2017 came and we saw ECMAScript 2017 - you can see the pattern that’s emerging. 2018 came and we saw the introduction of more features in ES2018. You’ll notice these yearly code names were designed to replace the old confusing name styles and changes to make understanding all of these versions (and all of this history!) a little bit simpler.
You can also follow the latest standard drafts on GitHub!
So, let’s talk about browser support. Several years have passed and even now not all browsers (older ones, not evergreen browsers) actually support ES2015, let alone ES2016, ES2017, ES2018 and beyond.
An “evergreen browser” refers to a browser that is automatically updated to newer versions of itself. With older browsers, a user had to download a new version each time a new release was published.
So how do we learn what we would call “modern JavaScript” and actually deploy fully functioning production code?
What is Modern JavaScript?
Let’s take a very simple line of ES2015 code, a constant variable declared with a const
:
const name = 'Ultimate Courses';
The above code will run in the majority of browsers that exist today, but with older browsers (such as Internet Explorer and earlier versions of things like Chrome and Firefox) const
doesn’t actually exist therefore a runtime error will be thrown. So, what do we do here? It sounds like we need a way to ‘convert’ any new code into older-style code that can run in old browsers - as well as the new ones!
Compiling with Babel
As the release of ES2015 was on the horizon, a tool was being developed that allowed you to input ES2015 code and it would output ES5 code (which, as we know, is supported by all modern browsers). This tool is called Babel.
You may have heard of Babel - it allows you to use new features introduced to the JavaScript language before they even arrive in browsers. Well, that was the case a few years ago and browsers have actually caught up dramatically since then… However, there are still a few holes in some browsers where functionality hasn’t been completed yet and never will be.
Babel offers extended browser compatibility by transforming the newer code that we write into “compatible” JavaScript that can run on older environments. This allows us to write fully future-proof code, which may be more advanced and more concise, to give us the best of both worlds. The JavaScript we write nowadays is typically best written with developer tools, in my opinion, and introduce a compile step (instead of traditionally writing JavaScript in a .js
file and uploading it to your server).
Using ES2015 features is made possible by Babel transforming our source code into something that older browsers can also understand. Babel actually used to be called “6to5” as it was an ES6 to ES5 transformer.
In our example above of using const
, this keyword doesn’t exist in older browsers and therefore is renamed to var
. This is then outputted as the production file which would then be uploaded to the server instead of the raw source file written in the newer version of the language. You can think of this new file as a compatible version which you would then deploy to your web server which would then be served on the site or application.
So after all of this history and development, it’s considered a best practice now to introduce a compile step in our JavaScript apps. This allows us to use the latest features and technology which is then automatically back ported to support in older browsers.
At some stage we can then throw away a tool such as Babel and all of our code will be 100% fully working in a native browser without any build step transformations to port it back to old style JavaScript. It’s likely we’ll still use a build step, but it might not involve transforming our JavaScript as much.
Babel also allows us to specify which cutting edge features we’d like to use and how far back, in terms of browser support, we should go when compiling and transforming our initial source code back to something older browsers can \understand.
JavaScript Engines in Browsers Explained
So now we understand more about the JavaScript language, and where it came from, we want to think about how we use it today and how computers allow us to run JavaScript in a web browser.
JavaScript that we write, to be executed by a web browser, is made possible by something we call a “JavaScript engine”. This enginelives in the browser and essentially executes our code. Let’s explore what a JavaScript engine is and why we should be aware of it, and what it does underneath.
JavaScript is deemed an interpreted language and in recent years the lines between what we call interpreted and compiled, when talking about JavaScript, are now somewhat blurred.
There are many JavaScript engines such as Chrome’s V8, initially built by Google for Google Chrome as an open source project. V8 uses something called a Just-In-Time compiler typically shortened as “JIT”. In order to obtain more speed, V8 translates JavaScript code into more efficient machine code instead of using a traditional interpreter.
This methodology explains all the debate on whether JavaScript is interpreted or in fact compiled. Technically the code is compiled by the browser - but it’s compiled like an interpreter. Doing so makes for even bigger performance optimisations. Either way, for us JavaScript developers, these are just things to know that exist and for us to consider.
What’s also amazing about JavaScript is that we now see it running in other environments, such as NodeJS - which you may already be aware of. NodeJS essentially took V8’s JavaScript engine and moved it outside of the browser, allowing it to run on other platforms. This now allows us to write JavaScript on both the frontend (in the browser) as well as the backend (on the server server) in just one language - JavaScript.
Earlier I briefly mentioned something that lives in our browser called the “Document Object Model” or the DOM.
To be a JavaScript developer we must first learn JavaScript the language.
To build web applications that talk to HTML and CSS, we need to learn not only the JavaScript language, but also this Document Object Model - which we now call the DOM.
The DOM is an API for HTML and XML documents, and it provides us a structural representation of the document so we can then interact with it via JavaScript. This might be to modify the contents of the document, or its visual representations.
The key point here is that JavaScript is a separate entity and can talk to the DOM via the API’s exposed to us. Don’t confuse the DOM and JavaScript!
In NodeJS, there is no DOM (yes, I know).
If you were to attempt to communicate with HTML and CSS you can’t - it doesn’t exist. The DOM is only present in the browser because that is the JavaScript environment.
In NodeJS there is an environment - but it’s a server side environment. There is no browser, therefore there is no DOM.
With JavaScript as the language and the DOM and its many APIs, there are definitely two major parts to learn. When we’re ready we’ll be visiting things such as the DOM its APIs and exploring the browser in further posts. This series, however, is going to give you those initial building blocks and foundations for you to understand the JavaScript language how to use it to its full extent so you can confidently write JavaScript to a very excellent standard.
This series will also include modern features and practices such as ES2015 and beyond to give you the latest and greatest of the language. I’m excited to take you through these next steps to fully mastering JavaScript!