In the HTML we write, we declare elements and pass values into attributes. In JavaScript, specifically in the DOM, we have properties available when querying HTML elements via the DOM.
“How do properties relate to HTML attributes?” In this article, we’re going to answer questions like this as well as others like “What is the DOM?” and “How is an attribute different to a property?”.
Table of contents
After reading this article, you’ll be primed with new knowledge on the DOM, HTML attributes and JavaScript DOM properties - as the two can be very similar yet different.
Let’s investigate the difference between Attributes and Properties.
What is the DOM?
In web applications we write, we have HTML. Once a browser requests your HTML page, it’s time for the DOM to spring into life!
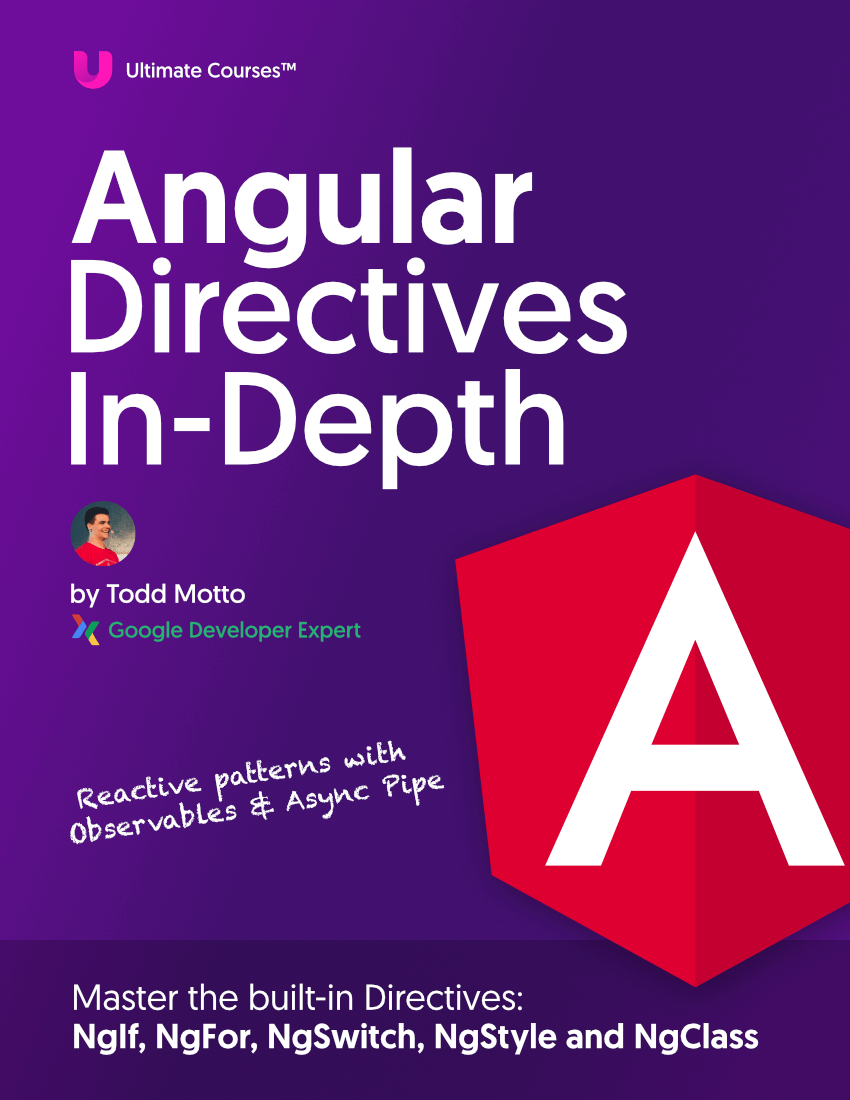
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Deep inside your browser, when a page is rendering, the DOM (Document Object Model) is created from the HTML source code that’s being parsed.
HTML is a structural language, and that structure (in short) gets mapped across to a JavaScript representation of that source code - ultimately giving us “the DOM”.
HTML is a tree structure, we deal with parent and child elements, so naturally the DOM is a JavaScript object following a similar structure.
For example, HTML such as <body id="ultimate">
would be composed into a DOM object which we could access via document.body.id
.
If you’ve done something like this, you’ve already used the DOM:
// returns a DOM Node object
document.querySelector('input');
The DOM gives us the ability to talk to different parts of our HTML structure, update them, create them, delete them - you name it. As the DOM is just a JavaScript object, all we need is knowledge of JavaScript to get started.
When our JavaScript code is executed by a browser, the DOM is ‘supplied’ to us from the environment we are running in, i.e. the ‘web’ environment. In a Node.js environment there is no DOM and therefore references to things like document
will not work.
JavaScript is the programming language, the DOM is an API exposed to us in the global scope, via the browser, for us to interact with.
With this in mind, we know how to write HTML and declare attributes. And we know how to query our HTML via the DOM in JavaScript. That is the succinct difference, and is just the beginning, as there is lots more to learn about attributes and properties - let’s continue.
Attributes versus Properties
In HTML there are attributes. In JavaScript DOM, we can talk to and update these attributes through properties (and even method calls) that exist on elements, known as DOM nodes, when we make a query. Let’s explore an attribute and then we’ll come onto properties.
An attribute could be something like value
, for example with this piece of HTML:
<input type="text" value="0">
When the above HTML is parsed and rendered, the attribute’s value will be '0'
and of type string, not number. What we’re interested in is the property. A property gives us access via JavaScript to read and write different values. Welcome to the DOM!
In plain JavaScript let’s query our input element:
const input = document.querySelector('input');
console.log(input.value); // '0'
When we access input.value
, we are talking to the property and not the attribute.
Now, if we want to update our value
, we use the property:
const input = document.querySelector('input');
// update the value property
input.value = 66;
// read the current value property
console.log(input.value); // '66'
Access and give this example code a try here.
This updates the DOM, our JavaScript model, and does not update the HTML. If we Inspect Element, we’d see value="0"
- yet the actual DOM value is 66
. This is because updating a property does not necessarily update an attribute, and this is a common gotcha for JavaScript developers of all skill levels.
If we wanted to actually update the HTML element’s attribute, we can do this via the setAttribute
method:
const input = document.querySelector('input');
// update the value attribute
input.setAttribute('value', 99);
// read the current value property
console.log(input.value); // '99'
Access and give this example code a try here.
The interesting thing here is our HTML would update from the setAttribute
method call, giving us an accurate reflection when we Inspect Element - however it also updates the DOM property too! Which is why input.value
is 99
when logged out.
Why the two? The DOM is a JavaScript model, so we don’t need to keep updating the HTML attributes, it could actually be a resource waste. If we only need to use the DOM, we should do so and not store state inside the HTML as well. The DOM is optimized and fast, so using something like value
over setAttribute
makes much more sense.