In this post you’ll learn a few ways to check a selected radio input (<input type="radio">
) and explore a few different options and approaches.
If you think using checked
is the only answer - it’s not!
We’ll answer questions such as “How can I check a specific radio button?” and “How do I check a radio button based off a value?”. Let’s go!
☕ Once you’re finished, learn how to Get the Value of Checked Radio Buttons!
Here’s the markup we’ll use to demonstrate setting a radio button’s value, notice how we’ll begin selecting a default value with HTML via the checked
attribute - that’s the first way to set a checked radio (and it works without JavaScript enabled too 😉):
<form name="demo">
<label>
Mario
<input type="radio" value="mario" name="characters" checked />
</label>
<label>
Luigi
<input type="radio" value="luigi" name="characters" />
</label>
<label>
Toad
<input type="radio" value="toad" name="characters" />
</label>
</form>
So now let’s get a reference to our form
as well as the group of radio
controls:
const form = document.forms.demo;
const radios = form.elements.characters;
Here we’re using the new HTMLFormElement.elements property which allows us to access the name="characters"
attribute on the input.
Note: All radio buttons that you’d like in the same group need to have the same
name="value"
attribute for them to “link together”.
So, how do we set the value and check the radio button? Let’s first explore setting the value pragmatically, through setting the value we want via a string, and secondly we’ll look at querying the specific radio to be checked and manually checking it.
Using the RadioNodeList.value property
If we log out the resulting value of const radios
we’ll see something interesting:
// ▶ RadioNodeList(3) [input, input, input, value: "mario"]
console.log(radios);
Well, that certainly looks interesting, a RadioNodeList? Nice!
The RadioNodeList interface represents a collection of radio elements in a
<form>
or a<fieldset>
element.
You’ll also note that this is an Array-like object, which means we can iterate it as well as access the .value
property - on the same object! We’re really only interested in this value
property which is the simplest way to set a radio button as checked if we have the value that we want to set:
const radios = form.elements.characters;
radios.value = 'luigi';
Here we’ll set 'luigi'
as the RadioNodeList.value
(the group of radios) which will mark the radio thats value matches as “checked” and we’ll see it update in the browser!
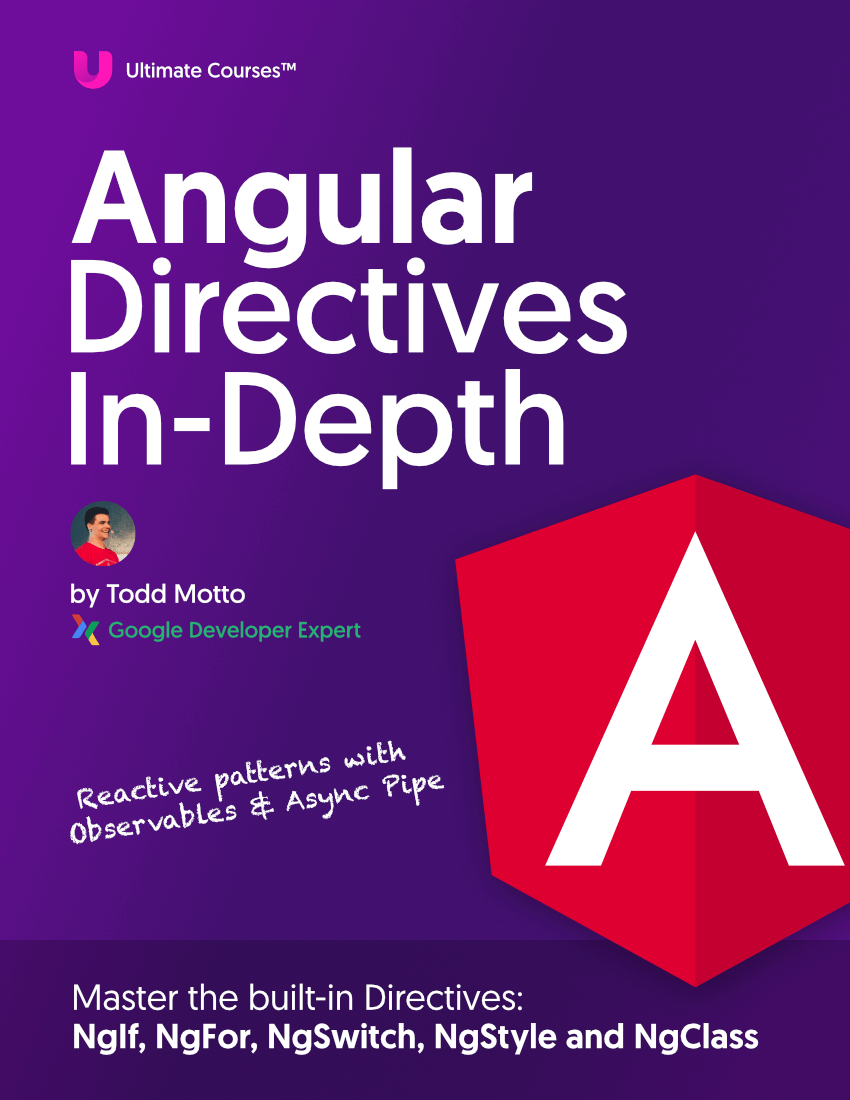
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
To demonstrate this technique more visually, we could combine an IIFE recursively use a setTimeout
to update the radios in our markup every 1 second, with a random value from our values
array!
👻 There’s something strange, that I just found out… using
radios.value
will set the value, however it won’t fire any “change” event listeners on that element. Who you gonna call?
const form = document.forms.demo;
const radios = form.elements.characters;
const values = ['mario', 'luigi', 'toad'];
(function selectRandomValue() {
radios.value = values[Math.floor(Math.random() * values.length)];
setTimeout(selectRandomValue, 1000);
})();
Try the live StackBlitz demo which demonstrates the recursive value randomiser:
So far we’ve used this radios.value
property, which gets us pretty much where we need to. However, it involves us knowing the value we want to set upfront, it might be that we want to somehow get a reference to a radio button and then set that radio button to checked.
Using the “checked” property
Now let’s look at the DOM Node level method of checking a radio button - the checked
property. It is as simple as setting it to true
!
First though, we need a reference to the DOM Node we’d like to set to checked
. As our radios
variable returns a RadioNodeList
, we could access its members like an array:
const form = document.forms.demo;
const radios = form.elements.characters;
const radioToUpdate = radios[0];
radioToUpdate.checked = true;
We could use radios[1]
or radios[2]
to get further values, and yes a NodeList
also has zero-based index system like an array.
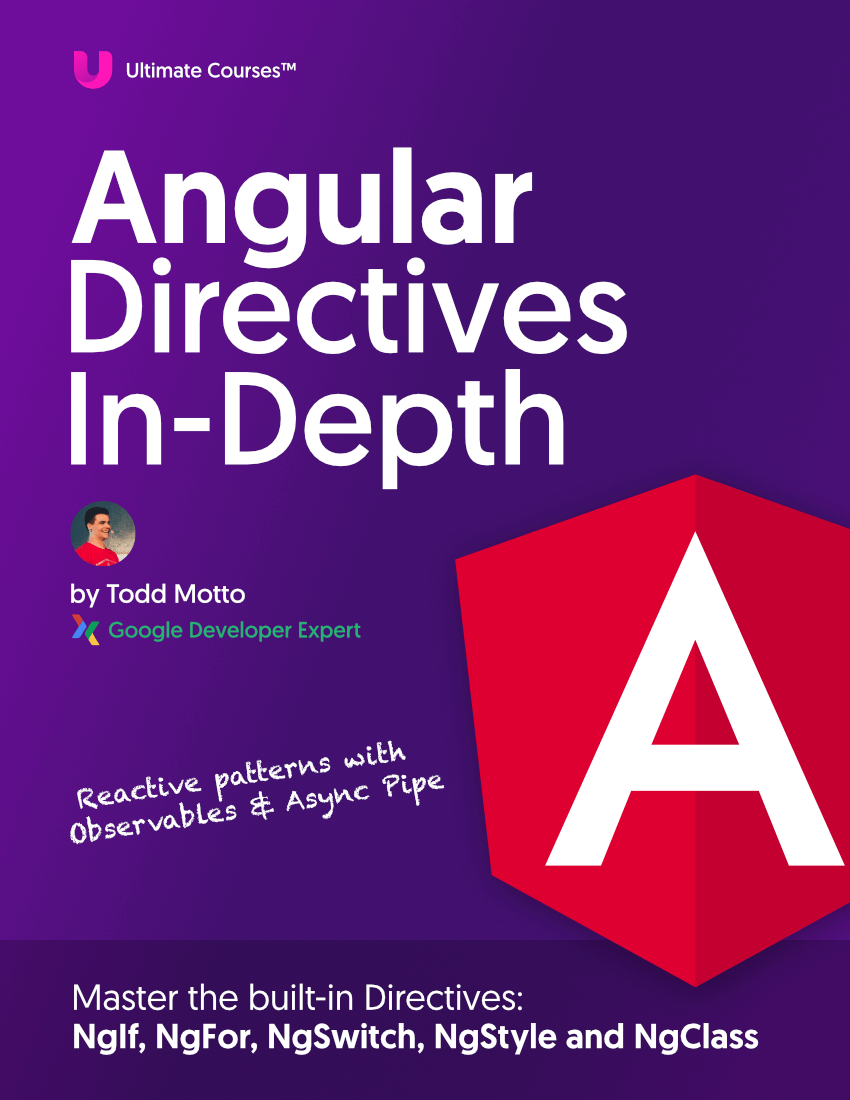
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
It’s worth mentioning that we can also randomly select a chosen index from our RadioNodeList
and set that radio button’s property to checked
:
const form = document.forms.demo;
const radios = form.elements.characters;
(function selectRandomValue() {
radios[Math.floor(Math.random() * radios.length)].checked = true;
currentValue.innerText = radios.value;
setTimeout(selectRandomValue, 1000);
})();
Here’s another StackBlitz example that also live updates each 1 second:
Summary
So many options! Which is the best one to choose? If you have a reference to that radio button, you might as well go ahead and use the checked
property.
If you’ve got a few values that also match some of the radio values, you can use the radios.value = 'luigi'
syntax that we explored - this is also really nice as you don’t need to deal with the DOM Node directly, we’re dealing with the RadioNodeList
wrapper that is holding the radios!
😎 Next up learn how to Get the Value of Checked Radio Buttons to complete your Input Radio knowledge!
Browser support
Check the browser support for:
tl:dr; the browser support in evergreen browsers is super.
The .elements
has no Internet Explorer support but has Edge support.
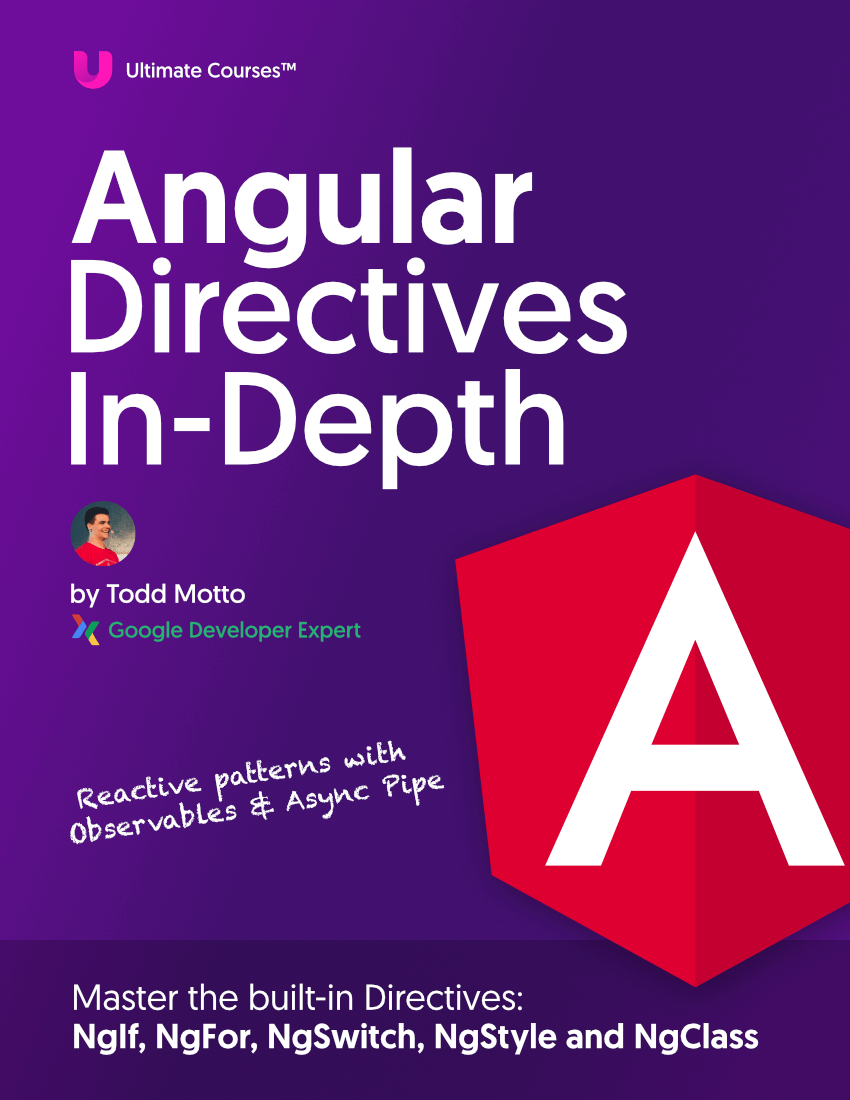
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
I hope you enjoyed the post, and if you’d love to learn more please check out my JavaScript courses, where you’ll learn everything you need to know to be extremely good and proficient at the language, DOM and much more advanced practices. Enjoy and thanks for reading!