JavaScript has come a long way in recent years, introducing some great utility functions such as Object.keys, Object.values and many more. In this article, we’ll explore how to check if a JavaScript Object has any keys on it.
First, let’s quickly demonstrate the “old way” of doing things, which would involve using a for
loop:
const item = { id: '🥽', name: 'Goggles', price: 1499 };
let hasKeys = false;
// oldschool approach
for (const key in item) {
if (item.hasOwnProperty(key)) {
// a key exists at this point, for sure!
hasKeys = true;
break; // break when found
}
}
You’ll notice we need to introduce the break
statement (don’t forget, we don’t want any bugs in our codebase), which leads us onto more modern, less error-prone approaches to use better tools.
The more modern and simpler approach, which also follows a Functional Programming style is to opt for Object.keys()
, which returns us an Array of the keys on an Object (who’d have guessed?):
const item = { id: '🥽', name: 'Goggles', price: 1499 };
// newschool approach
const hasKeys = !!Object.keys(item).length;
Combining this with a double-bang !!
we can convert the length
of the returned Array to a Boolean, ensuring we get a true
or false
reading. It’s not entirely necessary to do so, but I think the intent is more clear.
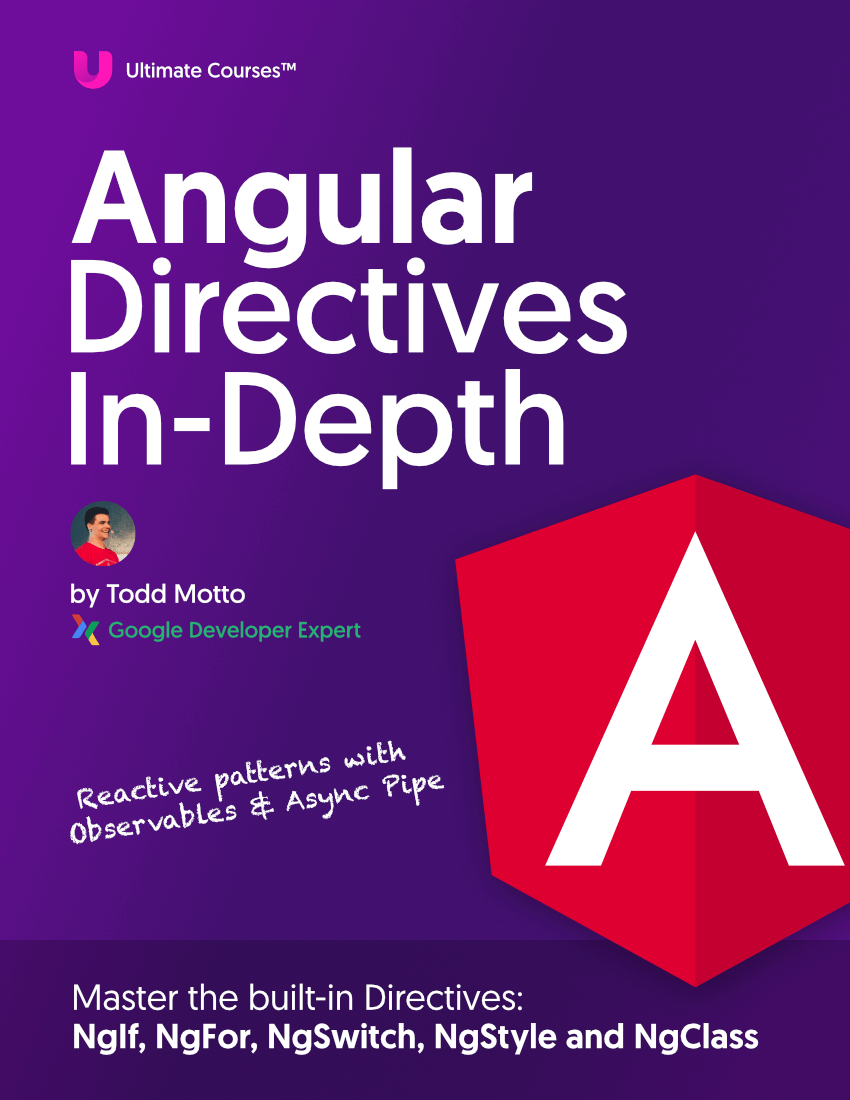
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
🎓 If you simply did
if (Object.keys(item).length) {}
it would still evaluate the same, but this relies on a “truthy” or “falsy” value. Sometimes it’s nice to have a straight-up Boolean and thus!!
is a nice quick way to get there. You could also useBoolean(Object.keys(item).length)
but that’s not nearly as cool…
If you wanted a reusable function to get the length of the Object’s keys, you could do something like this:
const item = { id: '🥽', name: 'Goggles', price: 1499 };
const getKeyLength = (x) => Object.keys(k).length;
const keyLength = getKeyLength(item); // 3
const hasKeys = !!keyLength; // true
🏆 Oh, and if you’d like to learn even more JavaScript, visit our JavaScript courses to see how they can help you level up your skills.
P.S. Here’s a StackBlitz embed with everything inside, so you can have a fiddle with it in real-time:
Thanks for reading, come check out our Newsletter to stay up to date with the latest and greatest in all things web.