With deno continuing to grow in popularity, one of the most common questions/requests I see regarding deno
is that there is an abundance of packages/frameworks/libs that have already been created for the nodejs
runtime to solve common problems, is there a way to utilize these libs in deno. In this post you’ll learn how to use packages from npm with Deno!
The deno
import pattern requires you to import ES Modules (ESM) with the included file extension, for example:
import { serve } from 'https://deno.land/[email protected]/http/server.ts';
Notice how the .ts
TypeScript file extension is included in the import path, this server.ts
file is an ESM.
Most NPM packages are not built and export in this way, yet, meaning they cannot be directly imported in deno
.
In this blog, I am going to show you one way to handle this problem. We are going to import the momentjs
lib as an ESM and utilize the lib to format a date and print it to the console.
Let’s talk Pika CDN.
To achieve this, we are going to use this incredibly awesome CDN called pika.dev. Pika is on a mission to make the web faster. That is a direct quote from their website:
We’re on a mission to make the web 90% faster
Pika hosts web-focused ESM packages in their CDN which is fantastic for us to use with deno
as this means we can import npm packages that we already know and use in our deno
runtime from Pika as an ESM.
This is incredible.
And, it even comes with TypeScript
typings and support as pika hosts type definitions (think like install a @types/
package from definitely typed) for every package where type definitions are provided to them. It handles this through the X-TypeScript-Types
header on the import so deno knows where to get to get the type definitions for the given package.
Using an NPM Package in deno
Lets use the Pika CDN to build a super simple deno
runtime app that will format and do some simple date manipulation.
To start, lets import momentjs
from Pika:
import moment from 'https://cdn.pika.dev/moment@^2.26.0';
Then, initialize a date object:
const today = new Date();
console.log('today is ', today.toDateString()); // Tue Jun 09 2020
Now lets use the imported momentjs
lib to format the date/time into a string with this format: MM/DD/YYYY hh:mm a
// initialise a moment instance from our date instance
const todayMoment = moment(today);
// format the moment instance with our given format string
const todayFormatted = todayMoment.format('MM/DD/YYYY hh:mm a');
console.log('today, but formatted, is ', todayFormatted); // 06/09/2020 08:47 pm
Look at that! We can now use the momentjs
package in our deno
app. Super easy. This was a great collaboration between the deno
and pika CDN
team to make this work.
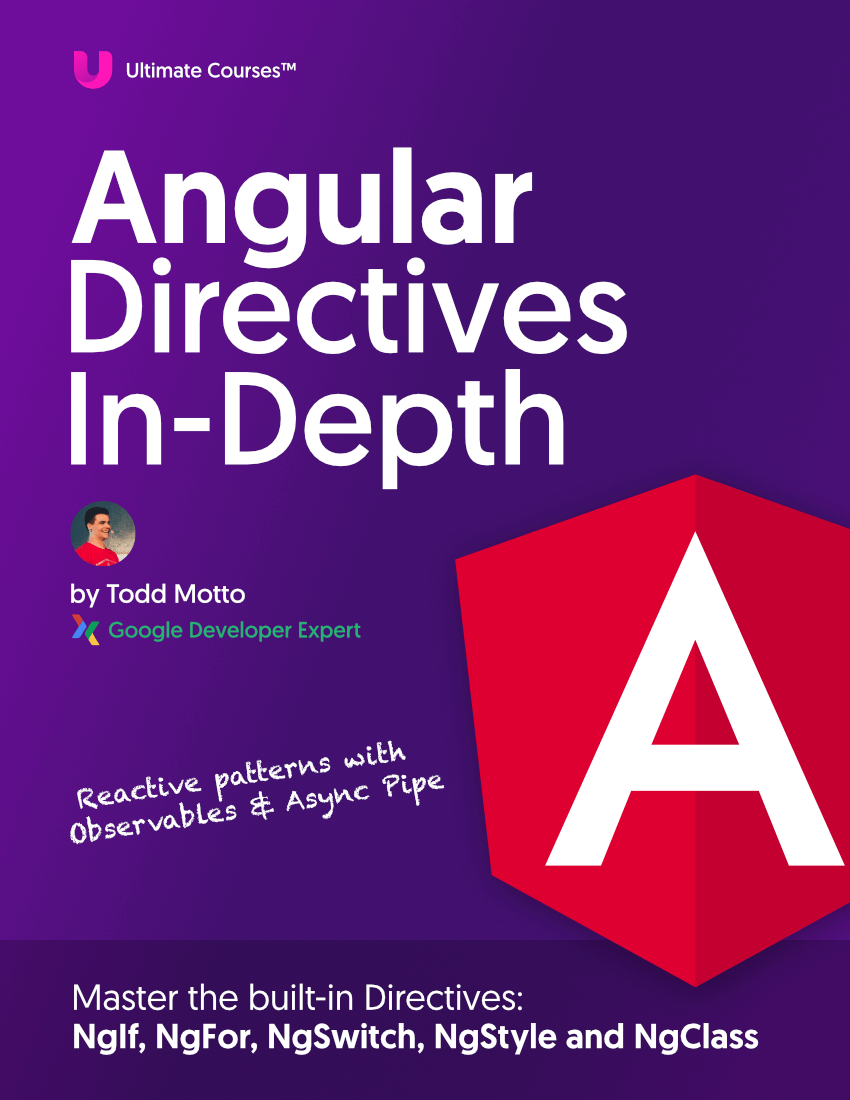
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Let’s manipulate our today
instance even more and use some more momentjs
functionality:
// add a day to the date to get to tomorrow.
const tomorrow = todayMoment.add(1, 'd');
console.log('tomorrow is ', tomorrow.format('YYYY-MM-DD')); // 2020-06-10
// get the first day of the current month from today
const firstDayOfMonth = todayMoment.startOf('month');
console.log(
'the first day of the current month is ',
firstDayOfMonth.format('YYYY-MM-DD')
); //2020-06-01
// get the last day of the current month from today
const lastDayOfMonth = todayMoment.endOf('month');
console.log(
'the last day of the current month is ',
lastDayOfMonth.format('YYYY-MM-DD')
); //2020-06-30
And that’s it! Now you’ve learned how to use an NPM package with deno, the world is your oyster.
🏆 Want to learn more JavaScript? Check out our JavaScript courses to fully learn the deep language basics, advanced patterns, functional and object-oriented programming paradigms and everything related to the DOM. A must-have series of courses for every serious JavaScript developer.
Thanks for reading, I hope you enjoyed the Deno article!