Let’s take an example String:
const location = 'Regent Street, London, England';
We want to search our String to see if 'London'
exists in it, and perhaps do something with it if there’s a match.
For this, we can use indexOf
(which exists on String.prototype
):
const index = location.indexOf('London');
// 15
console.log(index);
Here we’ve used indexOf('London')
to search our String for a match!
Our logged result is 15
, which means there was a match. The indexOf
method will always return -1
if there was no match. IndexOf always returns a number.
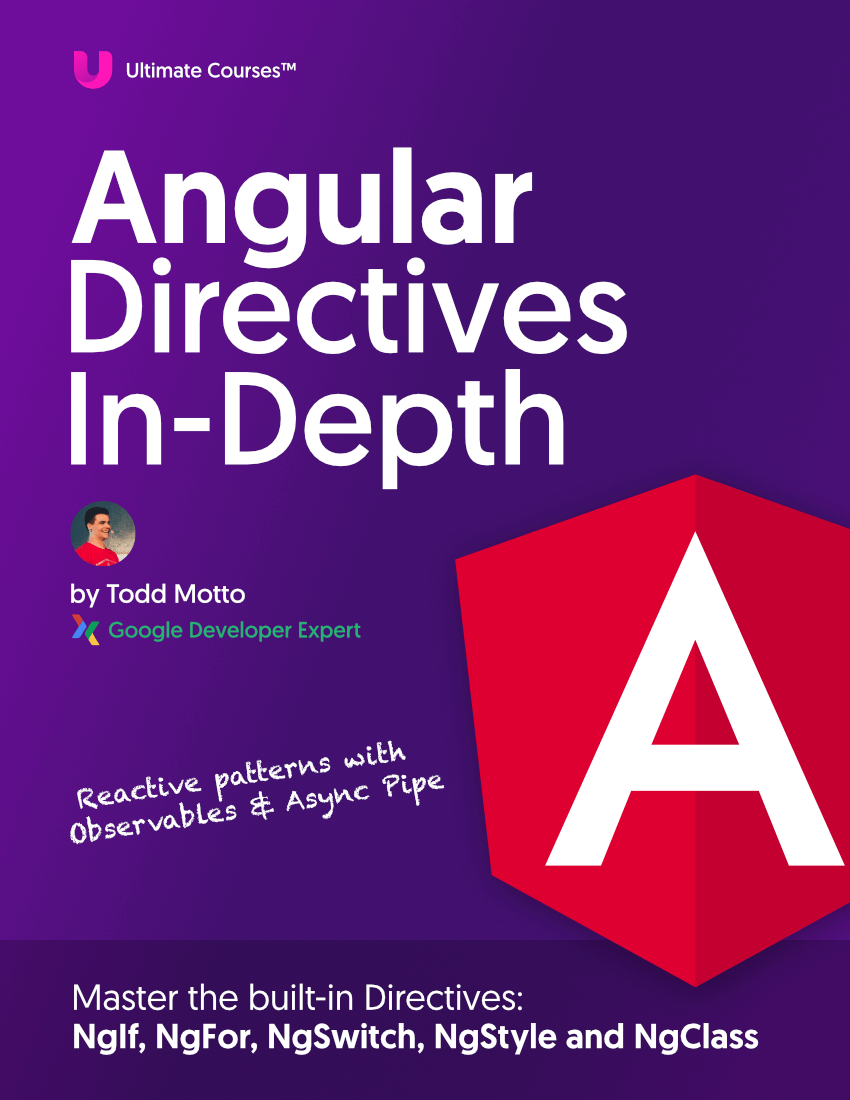
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
IndexOf allows us to search a string in JavaScript for a match.
So what is this number being returned to us? It’s the index of the first character’s position when there is a match. So how do we use it?
Typically we would use indexOf
combined with a conditional statement - but before we go there, consider this example:
const string = 'abcdef';
const index = string.indexOf('a');
// 0
console.log(index);
It returns zero! That means if we did this:
if (index) {...}
… and fatefully assumed this would work across all numbers, we would be greeted with a nice bug - because 0
is a falsy value whereas all other numbers, even negative, are truthy values.
To filter out this behaviour, and lock in some safety, it’s common that we’d do something like this:
if (index !== -1) {...}
You could also be more fancy and use the bitwise operator ~
which ensures any 0
values are coerced to -1
meaning they result in false
. This gives us a way of calculating whether something is true
- meaning _all indexOf
matches will now result to true
and -1
will result in false
- yay:
if (!!~index) {...}
Personally I would use the second approach on personal projects because I like the syntax, it’s clean and I get it. On a bigger project you may want to standardise usage with your team.
Altogether our code can look like so:
const location = 'Regent Street, London, England';
// 15
const index = location.indexOf('London');
// true
const existsInString = !!~index;
if (existsInString) {
console.log(`Yes, "London" exists in "${location}"`);
}
Give the live demo a try:
If you are serious about your JavaScript skills, your next step is to take a look at my JavaScript courses, they will teach you the full language, the DOM, the advanced stuff and much more!