Like all things JavaScript, there are many ways to accomplish the same task. Here we’ll dive into the various ways to check if a JavaScript string starts with something, such as a single character or multiple characters.
For the purposes of this article, we’ll be searching for the letter 'J'
at the beginning of the word 'JavaScript'
:
const word = 'JavaScript';
const char = 'J';
We’ll then use char
alongside a few different methods to demonstrate approaches to finding a character at the beginning of a string in JavaScript!
Based on what you’re doing, you might want to “extract” part of that string, however more often than not we actually need to check if the character(s) are even there to begin with. This means we’ll simply want a Boolean
(true/false) value back from our methods.
ES6 .startsWith()
Firstly, you’re probably here for the “correct answer”, so let’s start with String.prototype.startsWith
, a mildly new String feature introduced in ES2015 / ES6:
const word = 'JavaScript';
const char = 'J';
word.startsWith(char); // true
This is the most modern and recommended approach. The remaining methods in this article are simply for reference and different scenarios.
🕵️♂️ Note: This method isn’t supported in Internet Explorer, so you’ll need a polyfill and to check the browser compatibility.
indexOf
We can use String.prototype.indexOf
to return us the index of the particular character, so this one’s nice and simple:
const word = 'JavaScript';
const char = 'J';
word.indexOf(char) === 0 // true
However, unlike our good friend .startsWith()
you’ll notice that we have to do something with the return value and using === 0
to ensure that it fits the beginning of our string.
Of course as well, this method is just checking the first character, however will still work for multiple characters as it returns the index of the found item(s).
lastIndexOf
Before .startsWith()
arrived, this was the preferred solution as it was more performant.
Using String.prototype.lastIndexOf
allows the string to search faster as it begins at the index of 0
. It looks backwards through the string, starting at the beginning. Therefore this will get to the answer even faster. If it finds a match, it won’t search the entire string.
Similar to .indexOf()
we’ll also have to check the returned index using === 0
:
const word = 'JavaScript';
const char = 'J';
word.lastIndexOf(char, 0) === 0 // true
substring
An oldie but a goodie. Using String.prototype.substring
is also a nice method for fine-grained string control, as it allows us to specify a “search index” via two parameters. Start index, end index.
const word = 'JavaScript';
const char = 'J';
word.substring(0, 1) === char // true
You’ll notice that this doesn’t read as well as the other solutions listed above, and let’s be honest we all forget the difference between substring
and substr
and how to use them. MDN also considers substr
as deprecated, so I’ve chosen not to include it however using it in the same scenario as above will give the same results.
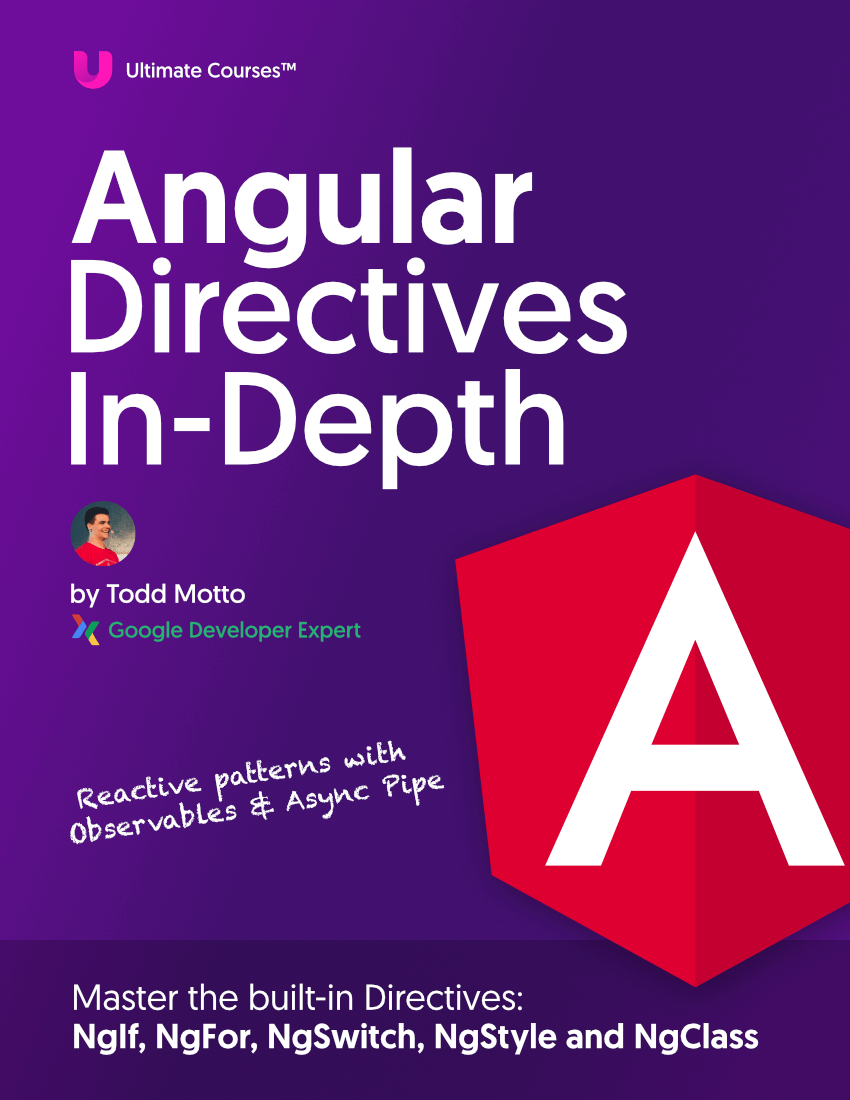
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
string[0] index
Similar to looking up “indexes” in an array, you can also use the square bracket syntax to lookup each “index” of a string. For example string[0]
would return the first item in the string, in the same way array[0]
returns us the first array item.
With this, we could access the initial character of the string, which requires no function call and should technically be one of the fastest mechanisms - though it does promote a little “oldschool” style code. These days, JavaScript is shifting towards a more functional style, with methods such as .startsWith()
amongst other utilities.
Here’s using the index lookup syntax:
const word = 'JavaScript';
const char = 'J';
word[0] === char // true
Regular Expressions
I love Regular Expressions (why, I hear you ask?!). I don’t know, they’re just great and make me feel like a “proper coder”. Nevertheless, let’s investigate some RegExp!
Built into JavaScript is a global function called RegExp
, which allows us to dynamically construct a Regular Expression.
Check out my in-depth guide to Regular Expression matching for more details on some
RegExp
prototype methods.
Here’s how we can use the ^
character (which means “the beginning of the string”) to check if our string starts with our character:
const word = 'JavaScript';
const char = 'J';
new RegExp(`^${char}`).test(word) // true
You’ll notice I’m using an ES6 string literal and some interpolation here. This is basically the same as doing new RegExp('^' + char)
but is obviously more awesome, as who likes concatenating strings anymore?
Finally, we could also “hard code” our Regular Expression and use it against .test()
(which returns a Boolean
, by the way):
const word = 'JavaScript';
const char = 'J';
/^J/.test(word) // true
But you can see how this would be less appealing.
All-in-all, you know startsWith
is the way to go, so what’re you waiting for?
🏆 Oh, and if you’d like to learn even more JavaScript you should come and check out my JavaScript courses. They’re the result of 10 years of developing and I’ve put every ounce of knowledge into them, I hope you find them super helpful for your career!
P.S. Here’s a StackBlitz embed with everything inside, so you can have a fiddle with it in real-time:
Thanks for reading, come check out our Newsletter to stay up to date with the latest and greatest in all things web.