Want to obscure an email address with JavaScript? Here’s how. Why might you want to do it? A bit of privacy!
We have lots of subscribers at Ultimate Courses, and I like to see a dashboard shared with my team members when new subscribers join. But, for privacy reasons, I of course want to protect their email addresses before our API sends them back. So, enter obscuring!
What obscuring allows us to do is take an email like this:
…and turn it into this:
t********@website.com
What I’m doing is replacing the number of characters in the email with an asterisk *
.
First, let’s create our function that accepts an email string:
const obscureEmail = (email) => {};
Now, we’ll use String.prototype.split
to split the email by the @
symbol.
A string split returns an array, and with modern ES6 features, we can infact easily destructure the “name” before the @
symbol and “domain” extension:
const obscureEmail = (email) => {
const [name, domain] = email.split('@');
console.log(name); // toddmotto
console.log(domain); // website.com
};
From here, we need to replace the name
with asterisks, but I want to keep the first character instead of obscuring it all.
To get the first character from name
we could use substring
or just a quick property lookup - as it’s shorter I’m going to vote for name[0]
.
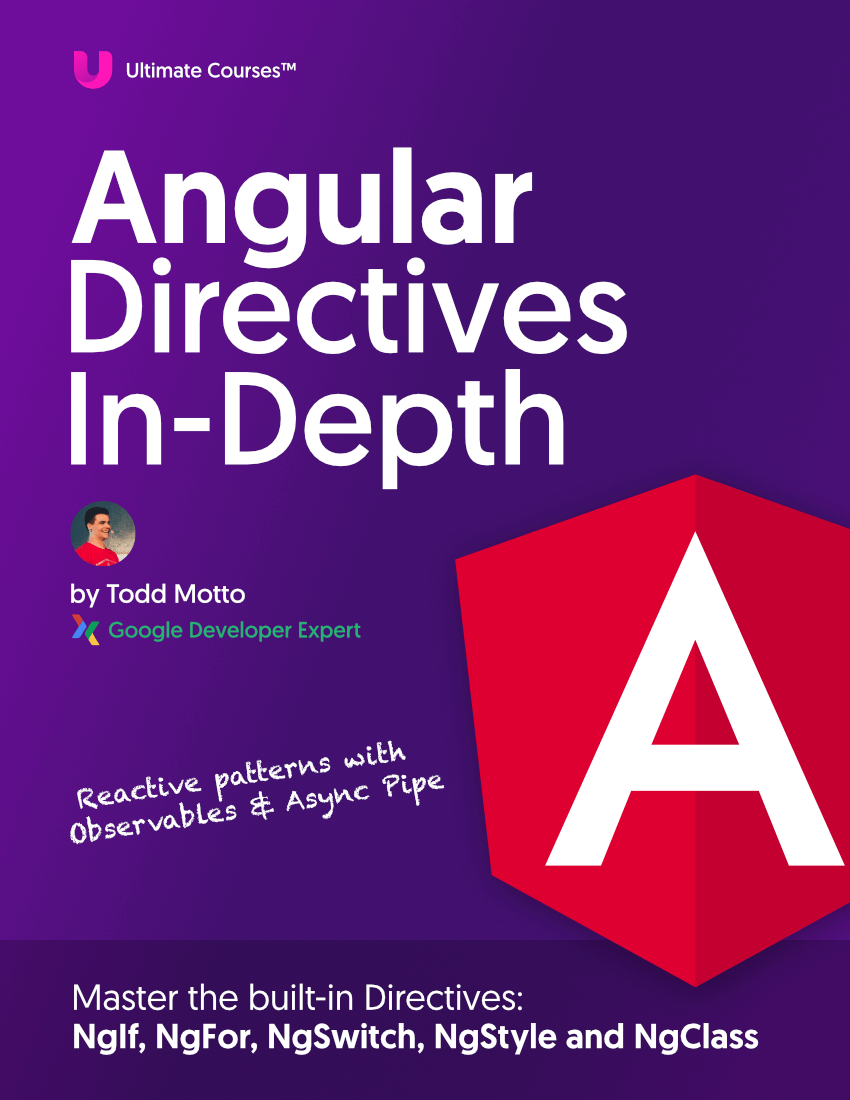
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
We can then cleverly use new Array(name.length)
to construct a new array from the length of the string, which will give us an array with undefined
values (we don’t care what’s in it, we’re going to join it):
[undefined, undefined, undefined, undefined];
Moving on, we can call new Array(name.length).join('*')
which will then join all 4 values and use 3 asterisks. Essentially, if you look above, each comma ,
will be replaced by *
a there are 4 items, there are 3 asterisks.
Then we can append the domain
and we’re done:
const obscureEmail = (email) => {
const [name, domain] = email.split('@');
return `${name[0]}${new Array(name.length).join('*')}@${domain}`;
};
const email = obscureEmail('[email protected]');
console.log(email); // t********@website.com
🏆 Oh, and if you’d like to learn even more JavaScript, visit our JavaScript courses to see how they can help you level up your skills.
P.S. Here’s a StackBlitz embed with everything inside, so you can have a fiddle with it in real-time: