In this post you’ll learn how to remove properties from an object in JavaScript using destructuring and the ...rest
syntax.
This new way to remove object properties (by excluding them from a new object) gives us a cleaner way to write JavaScript, with less code and better readability - as well as it being an immutable operation.
Before destructuring, we would typically use the delete
keyword to remove properties from an object. The issue with delete
is that it’s a mutable operation, physically changing the object and potentially causing unwanted side-effects due to the way JavaScript handles objects references.
By using object destructuring, combined with the rest operator ...rest
, we have a one-liner clean approach.
What is Object destructuring?
Object destructuring allows us to create variables from object property names, and the variable will contain the value of the property name - for example:
const data = { a: 1, b: 2, c: 3 };
const { a, b, c } = data;
console.log(a, b, c); // 1, 2, 3
By using const { a, b, c } = data
we are declaring 3 new variables (a
, b
and c
).
If a
, b
and c
exist as property names on data
, then variables will be created containing the values of the object properties. If the property names do not exist, you’ll get undefined
.
…rest in Object destructuring
First came rest parameters, then came rest properties!
Added in ECMAScript 2015 and now at Stage 4 - they’re here to stay and use today.
With this in mind, how does ...rest
help us remove a property from an object via destructuring?
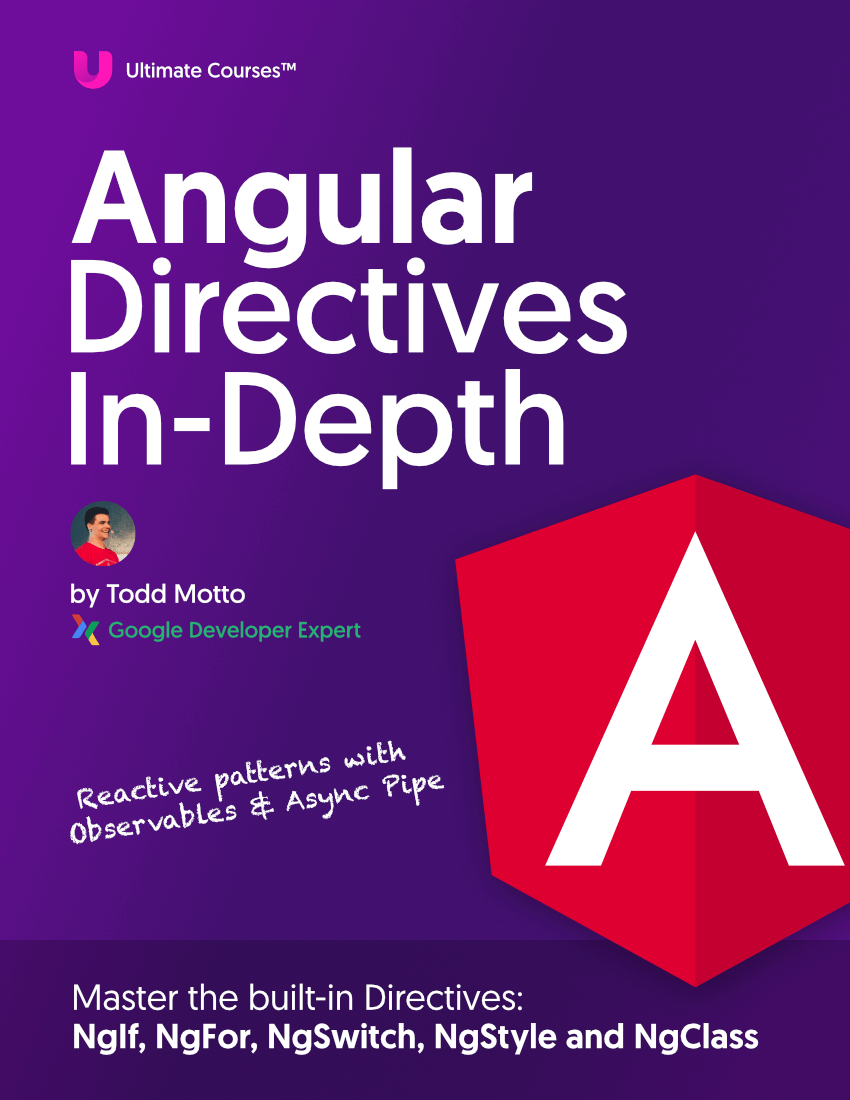
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Let’s take our earlier example and use the ...rest
syntax to see what happens:
const data = { a: 1, b: 2, c: 3 };
const { a, ...rest } = data;
console.log(a); // 1
console.log(rest); // { b: 2, c: 3 }
Using ...rest
here (and you can call rest
anything you like) gives us everything left over, the urmm “rest” of the properties if you will.
So, haven’t we just learned how to remove a property from an object? I don’t see a: 1
inside rest
- it’s been removed!
Technically, it’s been excluded rather than physically “removed” from the object - and it follows an immutable pattern for writing JavaScript and managing state (state being our data
).
Here’s how we would do this without object destructuring or the rest syntax, it’s a lot more tedious and doesn’t allow us to dynamically get all remaining properties:
const data = { a: 1, b: 2, c: 3 };
const a = data.a;
const rest = { b: data.b, c: data.c };
console.log(a); // 1
console.log(rest); // { b: 2, c: 3 }
Dynamically removing a property by key
Let’s say we have a key that perhaps a user has supplied that they want to delete. For this we’ll use b
. How do we remove that specific item? All examples so far have been hard coded values.
Let’s assume we have 'b'
as a value somewhere, we can dynamically pass this into our destructuring syntax using square brackets syntax [removeProp]
, just like an object lookup (instead this creates a new variable based off the dynamically passed value):
const data = { a: 1, b: 2, c: 3 };
const removeProp = 'b';
const { [removeProp]: remove } = data;
console.log(remove); // 2
That’s right, because we’re dynamically constructing a new variable, we need to use : remove
to reassign it a new name. Otherwise, how will we reference it? We can’t reference [removeProp]
so we’ll need to remember this.
However, at this point we can again introduce the rest syntax to remove the property from the object:
const data = { a: 1, b: 2, c: 3 };
const removeProp = 'b';
const { [removeProp]: remove, ...rest } = data;
console.log(remove); // 2
console.log(rest); // { a: 1, c: 3 }
There you have it, we’ve successfully deleted a property dynamically in JavaScript using object destructuring and the rest syntax!
Check out the live example in StackBlitz, have a play around and get comfortable with it:
Summary
We’ve come a long way! This technique helps us avoid mutable operations and the delete
keyword, giving us an immutable pattern for removing properties from objects in JavaScript.
We’ve used object destructuring and looked at how to combine it with the rest syntax to give us a clean solution to removing object properties.
🏆 Check out my JavaScript courses to fully learn the deep language basics, advanced patterns, functional and object-oriented programming paradigms and everything related to the DOM. A must-have series of courses for every serious JavaScript developer.
Happy coding!
P.S. this blog post was prompted by a neat discussion on Twitter I had with Mauricio Correa! He’s an awesome guy and developer from Brazil.
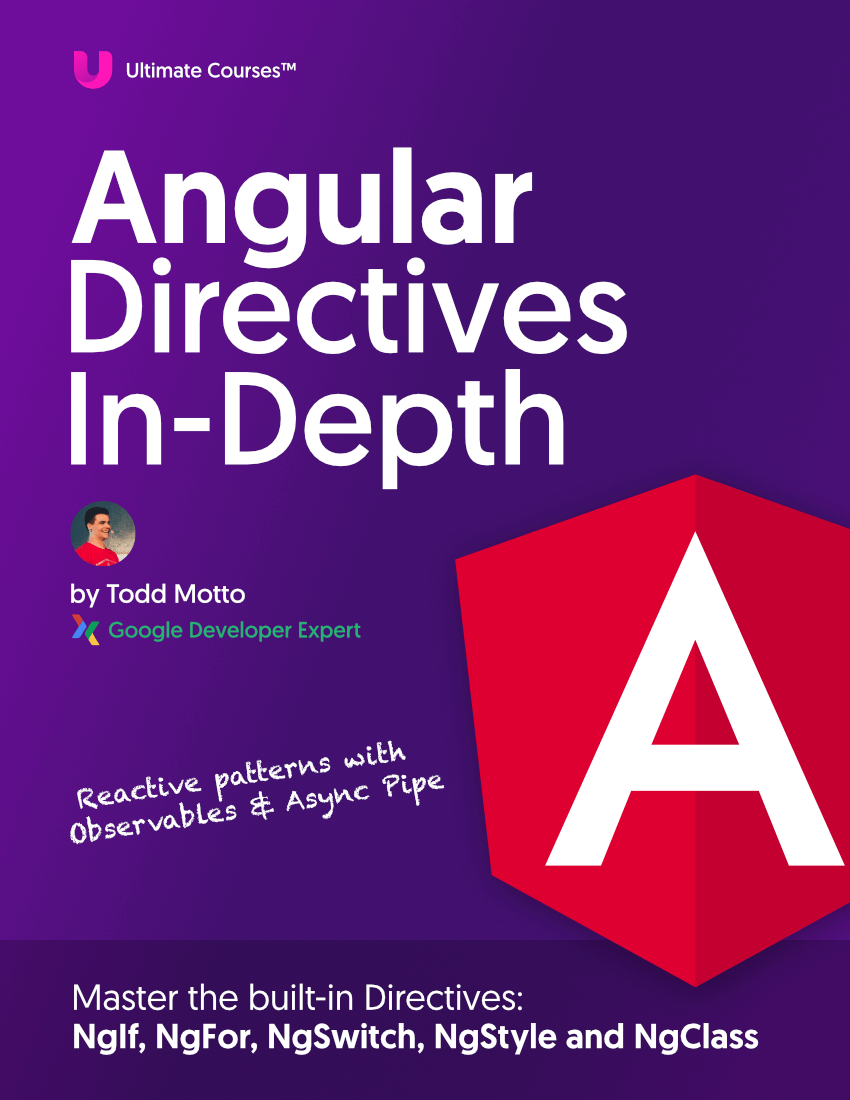
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings