In this article, we’ll explore a few different ways to remove an item from an array in JavaScript. I will also show you mutable and immutable operations so you know how your code will affect your data structures and references.
Removing items from arrays simply boils down to finding the index of the item, or at least traditionally. Nowadays - we have other options, such as finding items inside an array by their value. Which means there are now multiple approaches to removing items.
Table of contents
This next short section will dive into array indexes and how they come to be, it’s a nicer deep dive - but if you’re after the solutions then scroll right on through.
Understanding Array indexes
In JavaScript, an array is a special kind of object.
This array object has several different properties, some of which are inherited properties that exist on the prototype, and some are properties that the array object exposes to us when we “add” things to an array.
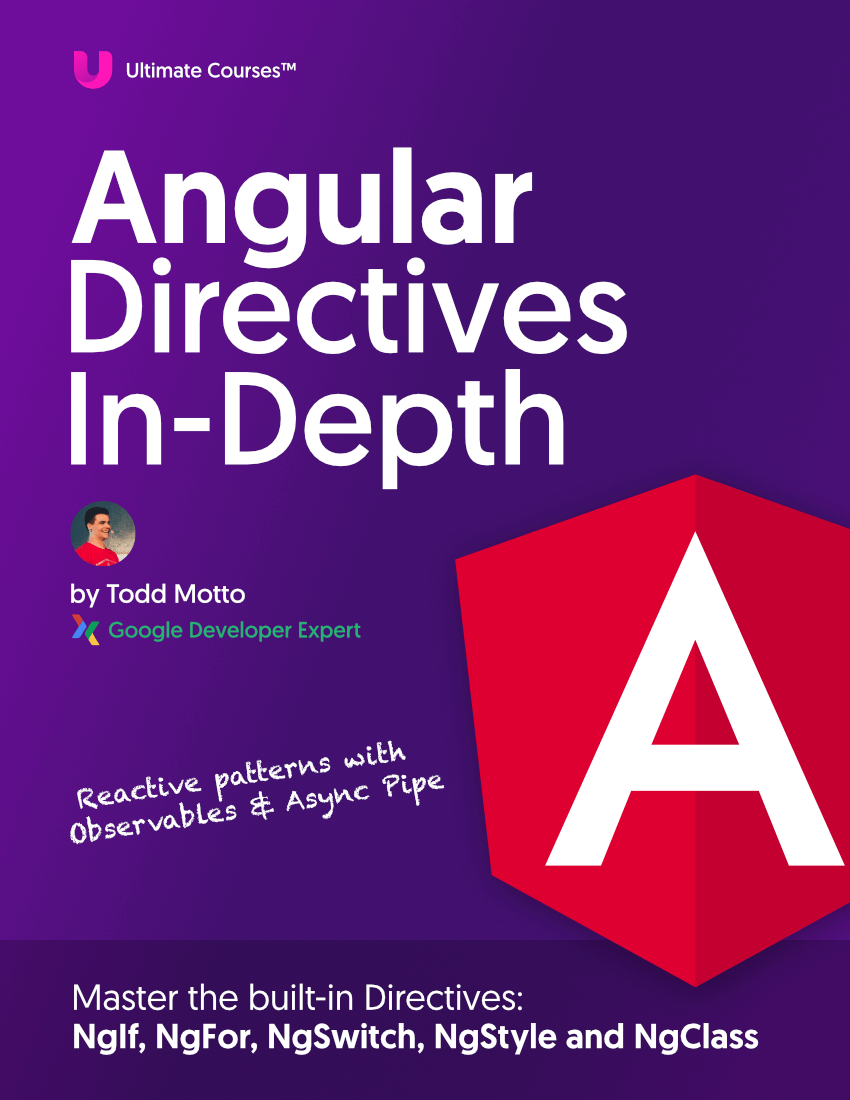
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
For example when we declare a new array via []
, that array contains Array.prototype
properties and methods that allow us to interact with our array. The intention with this was to allow us to perform mutations and changes to our arrays with the nice utility functions “built-in”.
A utility? Yes, for example Array.prototype.forEach() or Array.prototype.push(). Both of these are inherited methods on the array object. With that said, you now know how arrays are actually just objects - and objects have methods and properties.
The array object is special, it’s different. It is still an object, but the way that we “use” an array is by accessing properties on it that refer to an item in the array, via an index. An index? Yes - 0
, 1
, 2
, 3
and onwards! Array indexes start at zero.
With arrays this means when we need access to a value, whatever it may be (a primitive/object), it’s done via an “index lookup”. Ask for the index from your array and you’ll get back the item located at that index.
Really, you can think of each index as a drawer in a filing cabinet. Each draw contains something unique! Arrays are virtual, so the size of the filing cabinet is up to you!
“Why are you telling me all this, Todd?”
Because now you understand an array, you know how to work with one!
Without further ado, let’s first explore how to remove an item from an array in JavaScript by index and value.
Using .indexOf() and .splice() - Mutable Pattern
To remove an item from array via its index, we’ll first introduce the Array.prototype.splice
method and then investigate a better pattern using Array.prototype.filter
, a newer API.
Splice is a mutable method that allows you to change the contents of an array. This could be removing or replacing “elements”, as array items are known.
Let’s take an array with a few string values:
const drinks = ['Cola', 'Lemonade', 'Coffee', 'Water'];
To remove an item via its index, we need to find the index.
There are two scenarios here, 1) we know the index and 2) we don’t know the index but know the value.
First, if we know the index, below I’ve used id
to hold our example index, then it simply requires passing that index into .splice()
. The first argument of splice accepts the index position to begin removing items, and the second argument set to 1
represents the end - meaning we only want to remove one item after that index:
const drinks = ['Cola', 'Lemonade', 'Coffee', 'Water'];
const id = 2;
const removedDrink = drinks.splice(id, 1);
If we don’t know the index, then we need to find it. In this case, the index we’d like to remove contains a value of 'Coffee'
.
We could use Array.prototype.indexOf() to obtain the, well… “index of” the element:
const drinks = ['Cola', 'Lemonade', 'Coffee', 'Water'];
const id = drinks.indexOf('Coffee'); // 2
const removedDrink = drinks.splice(id, 1);
Array.prototype.indexOf() will return
-1
if the element is not found so I’d recommend a safety check around this.
You’ll notice I’ve also added const removedDrink
. This is because .splice()
returns us the removed item(s):
// ["Coffee"]
console.log(removedDrink);
// ["Cola", "Lemonade", "Water"]
console.log(drinks);
Try the code example for this below:
Using .filter() - Immutable Pattern
So now we understand a little more about mutable and immutable, let’s uncover the immutable pattern to “remove” an element from an array.
The best way you can think about this is - instead of “removing” the item, you’ll be “creating” a new array that just does not include that item. So we must find it, and omit it entirely.
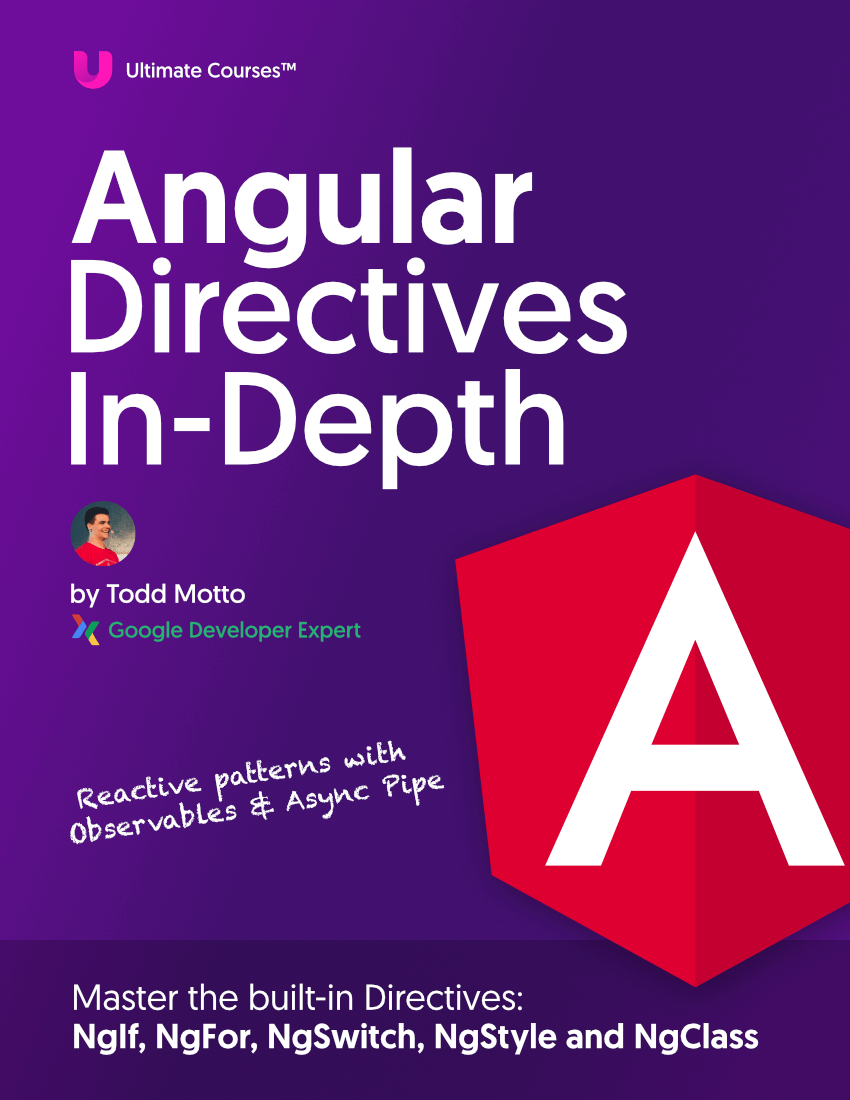
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
That last reference to your array will then only live on, and we won’t be mutating the original array. We’ll get back a fresh copy each time with our modifications!
So, let’s take the same array to start with:
const drinks = ['Cola', 'Lemonade', 'Coffee', 'Water'];
Again, let’s aim to remove 'Coffee'
. First, we could supply a value intended to represent an index value, and pass it into .filter()
:
const id = 2;
const removedDrink = drinks[id];
const filteredDrinks = drinks.filter((drink, index) => index !== id);
Note we included (drink, index)
as the function parameters and compared the array’s index
to the id
constant - so we can find the exact one we need as the indexes will match!
I’ve also included const removedDrink
which offers a way to “ask for” a reference of the drink you’d like to remove (in the same way you may want to use the return value from .splice()
).
If you haven’t got a reference to the index, for example our id
points to 'Coffee'
, then we can use .indexOf()
(please note the addition of the idx
variable to capture the index):
const drinks = ['Cola', 'Lemonade', 'Coffee', 'Water'];
const id = 'Coffee';
const idx = drinks.indexOf(id);
const removedDrink = drinks[idx];
const filteredDrinks = drinks.filter((drink, index) => drink !== idx);
Using a For Loop - Traditional Pattern
An alternate version, but a very valid version that really is the imperative version of Array.prototype.filter
as it behaves in the same way - constructing a new array for us and pushing new items in, leaving us an untouched drinks
array.
In this example, we are removing items from the initial array by returning a new array of just the items we do want, using drinks[i]
allows us to look at and compare the array element’s value (such as 'Coffee'
as a String in this case):
const drinks = ['Cola', 'Lemonade', 'Coffee', 'Water'];
const filteredDrinks = [];
const id = 'Coffee';
for (let i = 0; i < drinks.length; i++) {
if (drinks[i] !== id) {
filteredDrinks.push(drinks[i]);
}
}
Using filteredDrinks
would then give us the items we’d like. You can see how by learning the principles of programming we can apply different patterns and have deeper understandings of what we’re doing!
This next method I’ve included for clarity on what NOT to do when removing items from an array in JavaScript, even though it’s perfectly “valid”…
Avoiding the “delete” keyword
When I see the delete
keyword in codebases, I shudder a little - it’s a powerful keyword that should be used responsibly. That said, if we learn what the delete
keyword does, then we can make more informed decisions.
Here’s an example that you might see around the web:
const drinks = ['Cola', 'Lemonade', 'Coffee', 'Water'];
const id = 2;
delete drinks[id];
// Array(4) ["Cola", "Lemonade", empty, "Water"]
// 0: "Cola"
// 1: "Lemonade"
// 3: "Water"
// length: 4
// __proto__: Array(0)
console.log(drinks);
You’ll notice from the console.log
output above that it completely blows away the key. I included this for clarity, it’s always great to see and understand what side effects your operations have.
What’s more confusing is delete
doesn’t affect the Array’s .length
property - see how it still says length: 4
?! Another reason to avoid using delete
.
With that in mind - it’s time to conclude our post on how to remove an item, or “element”, from an array in JavaScript with the right tools. We’ve understood different approaches and paradigms and have some more knowledge to take to tomorrow. I thank you for reading!
If you love to learn and have a burning desire to level up your JavaScript skills? I teach JavaScript courses here at Ultimate Courses that will take your JavaScript skills to the max - I guarantee you won’t be disappointed!
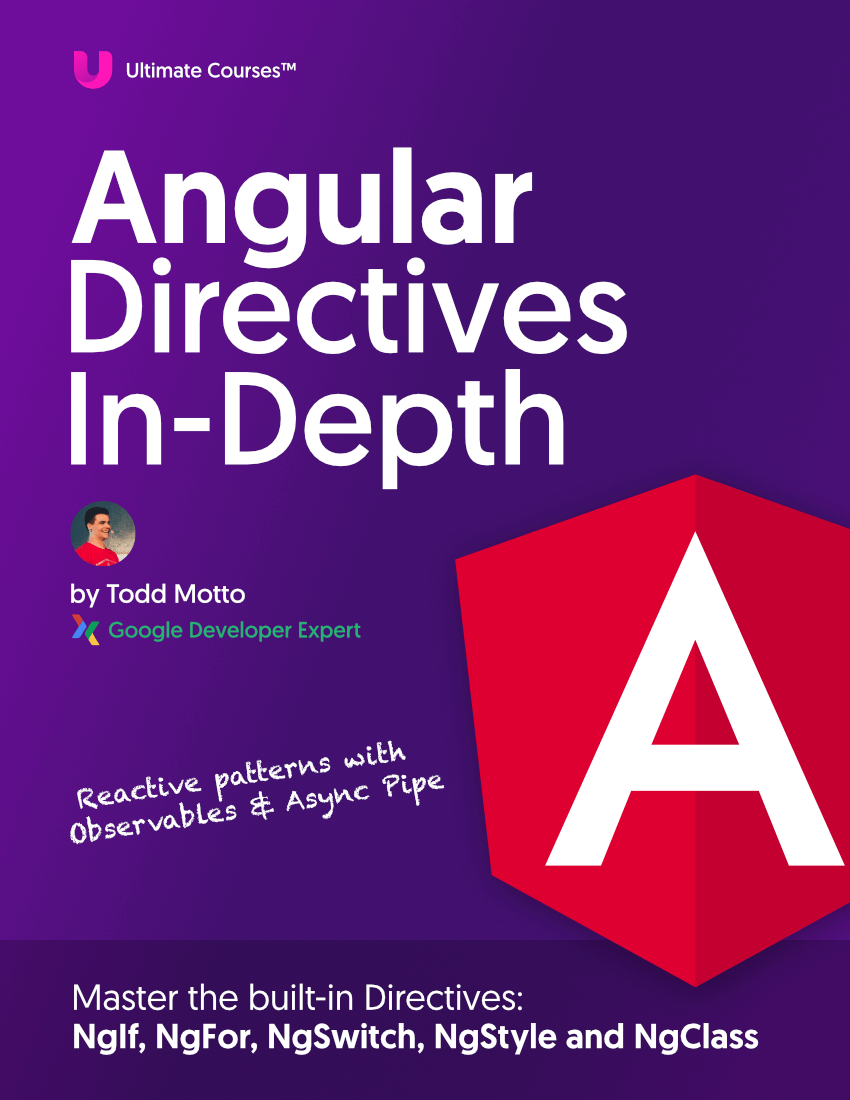
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Check them out and enjoy - thanks for reading!