In this post you’ll learn a few different ways to return an object from an arrow function. Sometimes you’ll just want to return an object and not use any local variables inside the function.
Let’s explore some examples that’ll neaten up your codebase and help you understand further workings of the JavaScript language.
The most common and standard way of returning an object from an arrow function would be to use the longform syntax:
const createMilkshake = (name) => {
return {
name,
price: 499
};
};
const raspberry = createMilkshake('Raspberry');
// 'Raspberry'
console.log(raspberry.name);
This pattern is great, as it allows us to easily add some local variables above the return
statement, a common practice for us.
But what if we don’t need to declare any local variables and just want to return an object?
We’ve heard of an arrow function’s implicit return feature - just delete the return
statement and the curly {}
braces right?
// ❌ Uncaught SyntaxError: Unexpected token ':'
const createMilkshake = (name) => {
name,
price: 499
};
And bam - a syntax error. This is what trips many developers up.
This is because the {}
we’re expecting to be the opening/closing object braces have now become the function curlies as soon as we remove the return
statement - the JavaScript parser acting as it should.
So, how do we solve it?
What’s interesting about JavaScript is its ability to create expressions using parentheses ()
. By doing exactly this and wrapping our intended object curlies in brackets, we create an expression and therefore return an expression.
This means that essentially the curlies are moved back “inside” the function and form the opening/closing object curlies once more:
// 👍 Perfect
const createMilkshake = (name) => ({
name,
price: 499
});
And that’s it. A really nice shorthand for returning objects from an arrow function.
Thankfully this ‘issue’ only applies to returning objects. For all other JavaScript types the implicit return works perfectly without this trick.
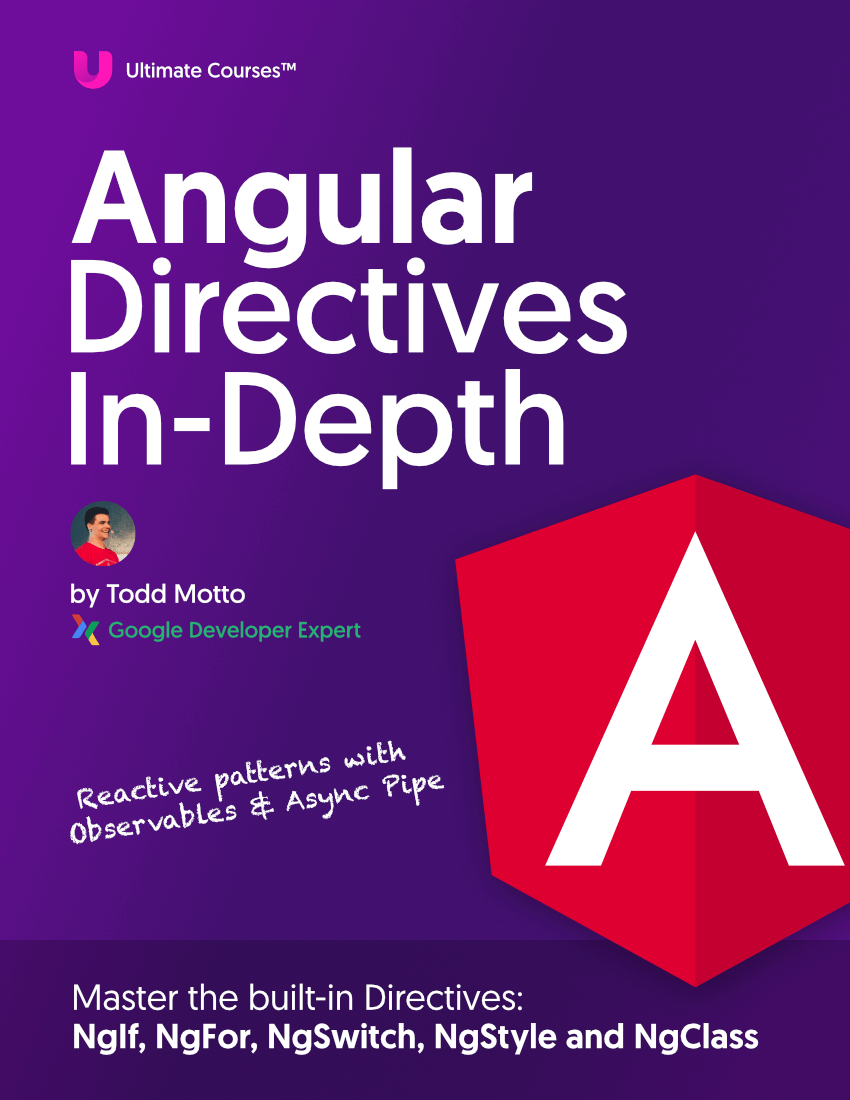
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
I hope you enjoyed the post, and if you’d love to learn more please check out my JavaScript courses, where you’ll learn everything you need to know to be extremely good and proficient at the language, DOM and much more advanced practices. Enjoy and thanks for reading!