JavaScript Objects are usually the driving force behind applications I develop, specifically JSON which gets sent back and forth from the server as acts as the main method of comms.
To save time rewriting the same (or similar) logic over and over again when dealing with our data (typically as part of a Model/View) - wouldn’t it be great to use one module to encapsulate the trickier object manipulation stuff and make developing the core of the application easier? It would also be great to bulletproof the object manipulation process, reducing object tasks, limit debugging, promote code reuse and even save a tonne of KB! Yes. So I built Stratos.js, a standalone 1KB module! It also comes fully equipped with unit tests for each method.
Table of contents
Stratos acts as a factory and supports: AMD (require.js), browser globals and module.exports
to run on Node/Browserify/CommonJS, so it can be used server-side too.
Stratos has a few helper utilities, as well as powerful and time/byte saving methods. The methods that Stratos currently ships with are:
- has()
- type()
- add()
- remove()
- extend()
- destroy()
- keys()
- vals()
- toJSON()
- fromJSON()
These methods take care of the heavy lifting that comes with Object manipulation, for instance to extend an Object, Stratos has a method that wraps it all up for you:
// "exports" is merely the inner module namespace
// you will call Stratos.extend(); in this example
exports.extend = function (parent, child) {
for (var key in child) {
if (exports.has(child, key)) {
parent[key] = child[key];
}
}
};
Another example of useful encapsulation; to delete object properties, Stratos makes the necessary safety checks as well:
exports.remove = function (object, key) {
if (exports.has(object, key)) {
delete object[key];
}
};
Stratos also has JSON support for stringifying and parsing Objects. Check out the rest of the methods and feel free to contribute.
Stratos runs in ECMAScript 5’s strict mode
, which I was interested to find out that you can’t delete Objects as a whole, so Stratos.destroy(object)
prevents Uncaught Errors
by emptying Objects rather than attempting to delete them entirely.
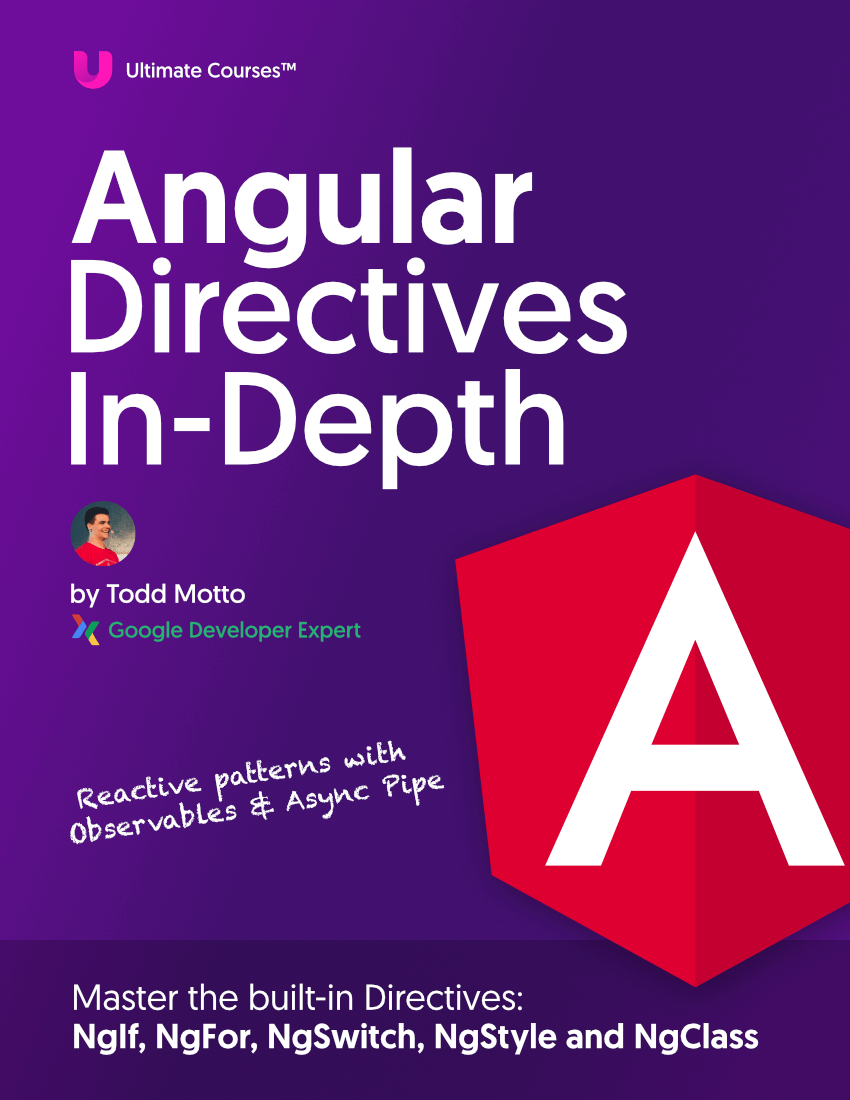
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
To ensure Stratos methods are called with the correct context, and do not conflict with other libraries or tools that override the hasOwnProperty()
method (which isn’t protected in JavaScript), Stratos uses Object.prototype.hasOwnProperty.call(object, key)
to ensure the correct context and method reliability.
Read on for a more in depth look into Stratos.
Methods/definitions
has()
Returns a boolean on whether an Object property exists.
var obj = { name: 'Todd' };
Stratos.has(obj, 'name'); // true
type()
Returns the raw type of the Object, for example [object Object]
.
var obj = {};
var arr = [];
Stratos.type(obj); // [object Object]
Stratos.type(arr); // [object Array]
add()
Adds an Object property with corresponding value. Value can be any Object type (array/number/object).
var obj = {};
Stratos.add(obj, 'name', 'Todd'); // { name: 'Todd' }
Stratos.add(obj, 'likes', ['Ellie Goulding', 'The Killers']); // { name: 'Todd', likes: ['Ellie Goulding', 'The Killers'] }
remove()
Removes an Object property.
var obj = { name: 'Todd', location: 'UK' };
Stratos.remove(obj, 'name'); // { location: 'UK' }
extend()
Merges two objects for top level keys. Stratos doesn’t offer a deep merge of Objects on a recursive basis.
var parent = { prop1: 'hello', prop2: 'yes', prop3: 'sing' };
var child = { prop1: 'goodbye', prop2: 'no', prop4: 'song' };
// { prop1: 'goodbye', prop2: 'no', prop3: 'sing', prop4: 'song' }
Stratos.extend(parent, child);
destroy()
Destroys an Object by removing all properties inside it, leaving an empty Object. ECMAScript 5 strict mode
doesn’t allow for top level Object deletion, so we will just erase the contents.
var obj = { name: 'Todd', location: 'UK' };
Stratos.destroy(obj); // {}
keys()
Traverses the Object and returns an array of the Object’s own enumerable properties, in the same order as that provided by a for in
loop.
var obj = { name: 'Todd', location: 'UK' };
Stratos.keys(obj); // ['name', 'location']
vals()
Traverses the Object and returns an array of the Object’s own enumerable properties, in the same order as that provided by a for in
loop.
var obj = { name: 'Todd', location: 'UK' };
Stratos.vals(obj); // ['Todd', 'UK']
toJSON()
Converts an Object to JSON.
var obj = { name: 'Todd', location: 'UK' };
Stratos.toJSON(obj); // {"name":"Todd","location":"UK"}
fromJSON()
Parses JSON back to an Object.
var obj = { name: 'Todd', location: 'UK' };
var json = Stratos.toJSON(obj); // {"name":"Todd","location":"UK"}
Stratos.fromJSON(json); // { name: 'Todd', location: 'UK' };
Thank you for reading!