In this post we are going to understand something called a variable. A variable technically means anything that is actually “variable” - something that can vary. Because JavaScript is a dynamically typed language it can hold absolutely any value, meaning it is truly a variable because it can be changed at any time.
Table of contents
Declaring variables
Let’s begin to create our first variable. For this JavaScript uses a reserved keyword called var
. We’ll take a look at reserved keywords momentarily because we need to know that we can’t create variables under the same name as things that are in fact a reserved keyword. So, for example I could create a variable called a and that could be the entire statement.
var a;
Now statements in JavaScript typically end with a semicolon as well. So this is a completely valid statement in itself. We have declared a new variable called a. But like I said what we can’t do is start creating variables called var
for example. That is a reserved keyword.
Now before we continue it’s good to know variables, unless they’re in different scopes which is something that will come on to later in this series to talk about scoping, all variables must have a fully unique name because otherwise they’re going to interfere and override each other. This is a common thing in many programming languages as well so if you’re coming from other languages it will be nice and familiar to you.
In JavaScript we have three ways to declare a variable. We have one the var
keyword which we’re going to learn about in this post. We’re going to explore how things such as hoisting works with variables and the two other ways are let
and const
which is a constant. But like I said they’ll be coming in the next few posts. So let’s talk about what we’ve actually done so far with our var a;
.
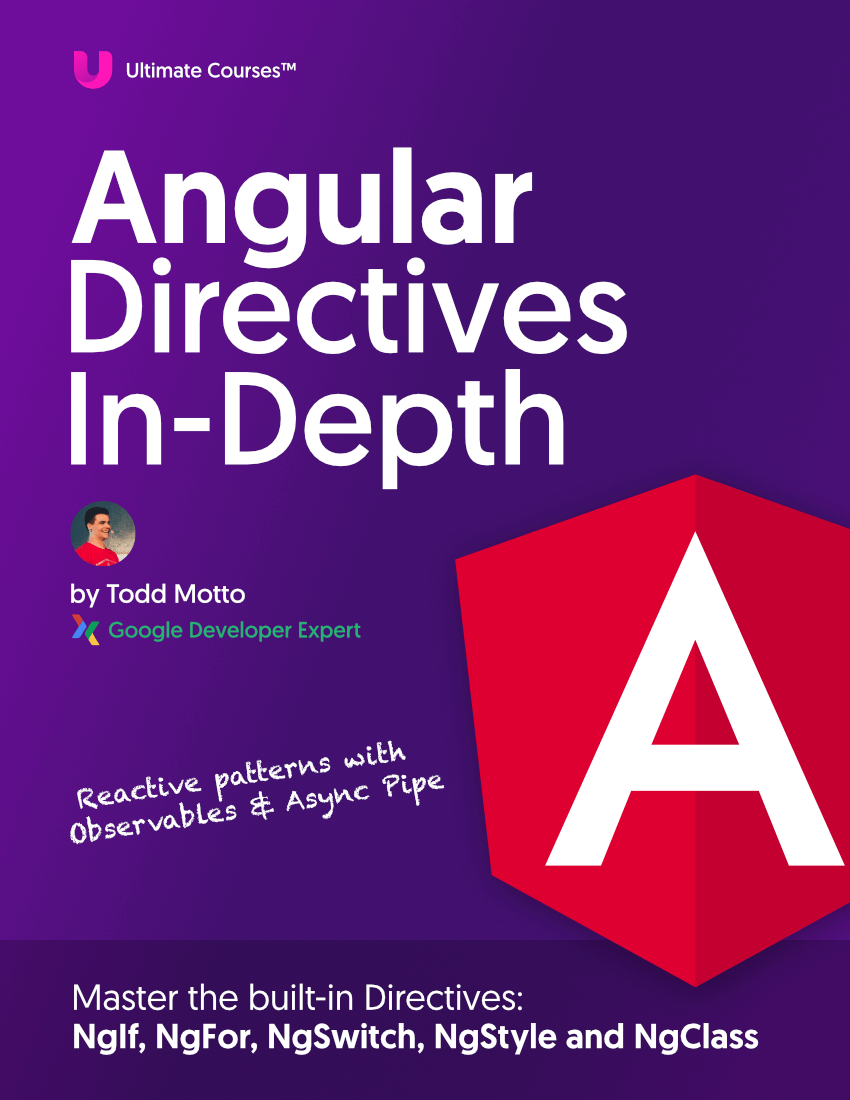
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
What we’ve done here is create something that we call a unique identifier. A is the identifier that we can go ahead and reference elsewhere. So for example we could say I want to console.log(a)
. This a
that we have created is actually called a declaration.
// declaration
var a;
console.log(a);
It is quite interesting the behaviour of a default variable declaration. If we were to save this out and look at our console we will see that the value will become undefined.
// undefined
Initialising variables
It’s interesting that there’s technically two parts to a variable and the first part of that is the declaration of the variable. So here we’re actually saying I’m creating this new identifier called a
and we simply just declaring it. The next step is initialisation. Now when we initialise a variable we then go ahead and give it a value. So here I’m gonna say that a is then going to be equal to the number ninety nine.
// declaration
var a;
// initialisation
a = 99;
console.log(a);
So when we go ahead and look at the console it will say 99.
// 99
The interesting piece is if you’re new to JavaScript this may surprise you: If we create two console logs we’re going to have undefined and then the number 99.
// declaration
var a;
console.log(a);
// initialisation
a = 99;
console.log(a);
// undefined
// 99
This is because we have created this variable here with no value, we’ve simply declared it. So at this point in time when our code is executed the first console.log(a)
we then see undefined and then in the second console.log(a)
we are initialising the variable with the number 99
. So when we reach our next line of code that then has the value that we want.
Something else about variables is we can actually declare multiple variables on a single line. So what we could say is we want to create b
it’s going to be the number 11
and we’ll have c
equals 55
.
// declaration
var a, b, c;
console.log(a);
// initialisation
a = 99;
b = 11;
c = 55;
console.log(a, b, c);
If we then went and logged these out in the console you can see the same thing will happen and we get our nice numbers. This demonstrates that you can comma separate from the variable keyword.
// undefined
// 99 11 55
However I don’t really like this pattern as it’s not very clear and people often put them on new lines and it can get confusing so my typical rule is if you’re creating multiple variable declarations I would recommend doing it something like this:
// declaration
var a;
var b;
var c;
console.log(a);
// initialisation
a = 99;
b = 11;
c = 55;
console.log(a, b, c);
Where it’s very easy to read and we can skim down the left hand side and we can see var
var
var
and then abc. So far we’ve looked at declaration and initialisation of variables but we can actually kill two birds with one stone and do them both at the same time. So we can say var a
equals 99 and we can change b
over to 11
and finally c
over to 55
. Then we edit our console.log(a)
to console.log(a, b, c);
.
// declaration
var a = 99;
var b = 11;
var c = 55;
console.log(a);
// initialisation
a = 99;
b = 11;
c = 55;
console.log(a, b, c);
and in our console we get:
// 99 11 55
// 99 11 55
Now what’s actually interesting is this first console log. Does in fact correspond to these values. Whereas this second console log we’re still technically overriding these variables. So if I went and changed a
to 66
:
// declaration
var a = 99;
var b = 11;
var c = 55;
console.log(a);
// initialisation
a = 66;
b = 11;
c = 55;
console.log(a, b, c);
We would see that change reflect in the console.
// 99 11 55
// 66 11 55
So this is what it’s meant by Javascript being a dynamically typed language we can assign dynamic types to it at any point in the program’s execution. This isn’t technically a dynamic type because it’s still a number. However we could change 66
over to be an object, we could change our second variable to be an array and finally we could change our third variable to be a string with the number '55'
inside.
// declaration
var a = 99;
var b = 11;
var c = 55;
console.log(a);
// initialisation
a = {};
b = [];
c = '55';
console.log(a, b, c);
// 99 11 55
// {} [] "55"
Now if you look at this, we then have our abc above with the respective values being logged out and then we’ve essentially overwritten them below. So JavaScript variables give you that opportunity to override them, reassign new values and we’ll look at let and const in the next couple of posts to understand some of the differences between these approaches when declaring different types of variables.
Variable hoisting
The next thing that I want to discuss is something called variable hoisting, which is an interesting topic and it kind of plays into what we’ve done so far. So with our existing knowledge in mind we’re gonna create a new variable called a
And will simply assign it the value of ninety nine. Now this is really great and we’ve learned so far the a
variable declaration such as giving it a name, an identifier of a
gives us a default value of undefined.
var a = 99;
What happens if I try and reference a variable that technically doesn’t exist yet and when we know that it doesn’t exist because JavaScript executes in order. So if I tried to console.log(a)
before declaring the variable like this:
console.log(a);
var a = 99;
// undefined
we would in fact get a different result as if I console logged it after:
var a = 99;
console.log(a);
// 99
So you can see here that we have the first console log gives us undefined
. And the second one gives us the number 99
. Now this is interesting because of the way that the declarations and the value of the variables actually work. So here the interesting thing to remember and this is quite a crucial thing to remember is the variable itself is hoisted and hoisting is essentially bringing the variable name to the top of the script declaration. So what the JavaScript engine is technically doing is this for us and then further down it’s reassigning it. So when we understand it this way, we have the value of undefined to begin with and then we are initialising that variable with the value of 99
. This typical behaviour where we have this variable which is magically put to the top is called hoisting in JavaScript.
That is a quick introduction and a worthy introduction to JavaScript variables how they work what hoisting is, what is in fact a declaration and an initialisation. And as we continue through this series we’ll be using many variables to hold different types of data. We’ll be logging them would be mutating data and so forth. So with that out he way. We’re going to have a look at something called let
the let statement in JavaScript in the next video.