React Router provides the <NavLink>
element for us to declaratively navigate around our applications, it renders an <a href="">
for us that points to a route. So, how do we add an active class using React Router when that <NavLink>
is active?
✨ Written for React Router v6, check out my brand new React Router v6 course to fully master it.
Thankfully adding an active class in React Router v6 proves nice and simple once we dive in. Our <NavLink>
component provides an isActive
property that contains a boolean value, which is exposed to us through the className
attribute when we pass in a function.
📣 You can also add active inline styles with React Router, the approach is similar so check it out!
From here we can an active class, or multiple classes, based on the isActive
value. We can also supply inactive classes for when the link is not active, perhaps to remove styles.
🚀 The “inactive” class feature was added into
<NavLink>
during React Router v6’s beta phase and shipped with thev6.0.0
stable release.
Let’s take a basic <NavLink>
which intends to navigate us to /users
when clicked:
<NavLink to="users">Users</NavLink>
To add an active class, we can use the className
attribute and pass in a function:
<NavLink
to="users"
className={() => console.log('I am called every route change...')}
>
Users
</NavLink>
This function gets invoked on every route change, which makes it the perfect place to add an active class (or add an inactive state).
When supplying a function, we’re given an object through the function arguments that contains an isActive
property:
<NavLink
to="users"
className={(state) => console.log(state)} // { isActive: true }
>
Users
</NavLink>
Presumably it’s an object in case further properties are introduced down the line.
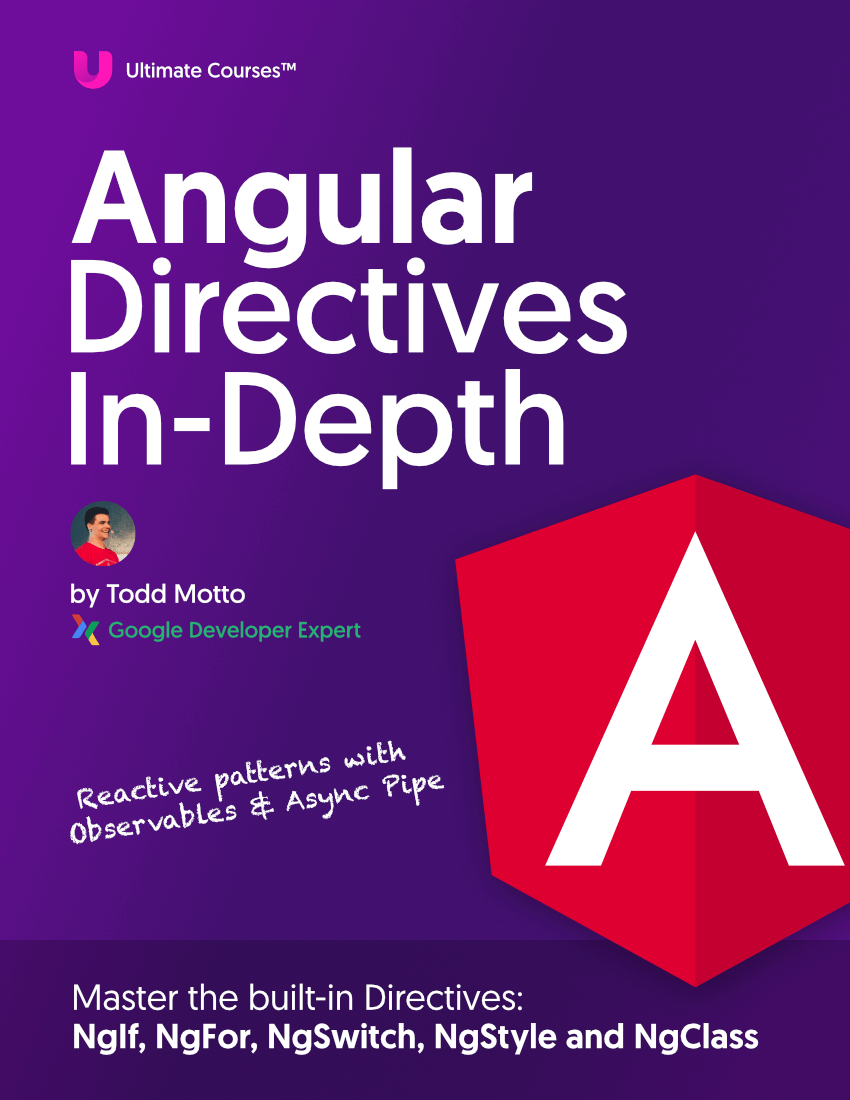
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
With this in mind, we can first destructure the isActive
value to clean things up and secondly declare the active class for our <NavLink>
in React Router as a string:
<NavLink
to="users"
className={({ isActive }) => (isActive ? 'active' : 'inactive')}
>
Users
</NavLink>
Yep, it’s that simple! If the <NavLink>
is active our 'active'
string is returned, otherwise it’ll fall back to 'inactive'
. You can call these classes whatever you like, and add as many as you like.
Check out the StackBlitz demo to see the React Router active classes on our <NavLink>
in action:
What’s more, this approach is also fantastic as it supports multiple classes (I’m looking at you, Tailwind CSS):
<NavLink
to="users"
className={({ isActive }) =>
isActive ? 'bg-green-500 font-bold' : 'bg-red-500 font-thin'
}
>
Users
</NavLink>
Nice and clean! And that’s pretty much all there is to it.
💯 If you are serious about your React skills, your next step is to take a look at my React courses where you’ll learn React, React Router, Styling and CSS practices, advanced concepts and APIs as well as server-side rendering and much more.
Happy routing!