Reading Query Strings, otherwise known as Search Params, from the URL is a common practice in web application development and you’re going to learn how to read them with React Router. React Router v6 provides a useSearchParams()
hook that we can use to read those query string search params that we need from the URL.
✨ Written for React Router v6, check out my brand new React Router v6 course to fully master it.
We can read a single query parameter, or read all of them at once, and we’ll also investigate a few other options.
I’m going to teach you a few different practices and concepts that you’ll need to handle reading search params from the URL, which involves a one-time single read as well as keeping an eye on any param changes via a useEffect()
React hook.
Get a single Query String value
Reading from the URL and getting your query string with React Router v6 is relatively simple but takes a few steps, and then you need to decide whether you want a single query string parameter or all of them at once.
First you’ll need to import the useSearchParams
hook and destruct the return value to get the current value of, React Router v6 calls these the “search params” due to them existing on the window.location.search
object:
import React from 'react';
import { useSearchParams } from 'react-router-dom';
const Users = () => {
const [searchParams] = useSearchParams();
console.log(searchParams); // ▶ URLSearchParams {}
return <div>Users</div>;
};
Logging searchParams
directly gives us a URLSearchParams
object, which is quite difficult to inspect the values by default due to the way it’s composed and the underlying Symbol.iterator
(which we’ll save for another time).
The URLSearchParams
object has a few methods that we can use to get a single value, the .get()
method.
If we assume this query string in the URL we can take a further look:
?sort=name&order=ascending
To get the sort
property value of name
from the query string, we can simply use .get('sort')
which is nice and easy:
const Users = () => {
const [searchParams] = useSearchParams();
console.log(searchParams.get('sort')); // 'name'
return <div>Users</div>;
};
This approach is all you’d need if you need to just read one or a few params, however there might be a better approach if you need to get all query string params at once.
Get all Query String values as an object
Next up is how to get all the parameters from the query string URLSearchParams
object. Thankfully we have an .entries()
method to use, however it doesn’t quite work out of the box if we want a straight up object to use, see below how it logs out Iterator {}
:
const Users = () => {
const [searchParams] = useSearchParams();
console.log(searchParams.entries()); // ▶ Iterator {}
return <div>Users</div>;
};
This means that we can… iterate… it. For example:
const Users = () => {
const [searchParams] = useSearchParams();
for (const entry of searchParams.entries()) {
console.log(entry);
}
return <div>Users</div>;
};
But this then logs out two arrays with a key as the first index and value as the second index:
['sort', 'name']
['order', 'ascending']
We could use some array destructuring to get the param name and the value:
const Users = () => {
const [searchParams] = useSearchParams();
for (const entry of searchParams.entries()) {
const [param, value] = entry;
console.log(param, value);
}
return <div>Users</div>;
};
Which then gives us a string to work with, so that works out nicely. So we now have two options, using .get()
or .entries()
, but neither approach gives us an object. We could compose one using our for...of
loop but that seems like extra work. Maybe there’s a better way? There is!
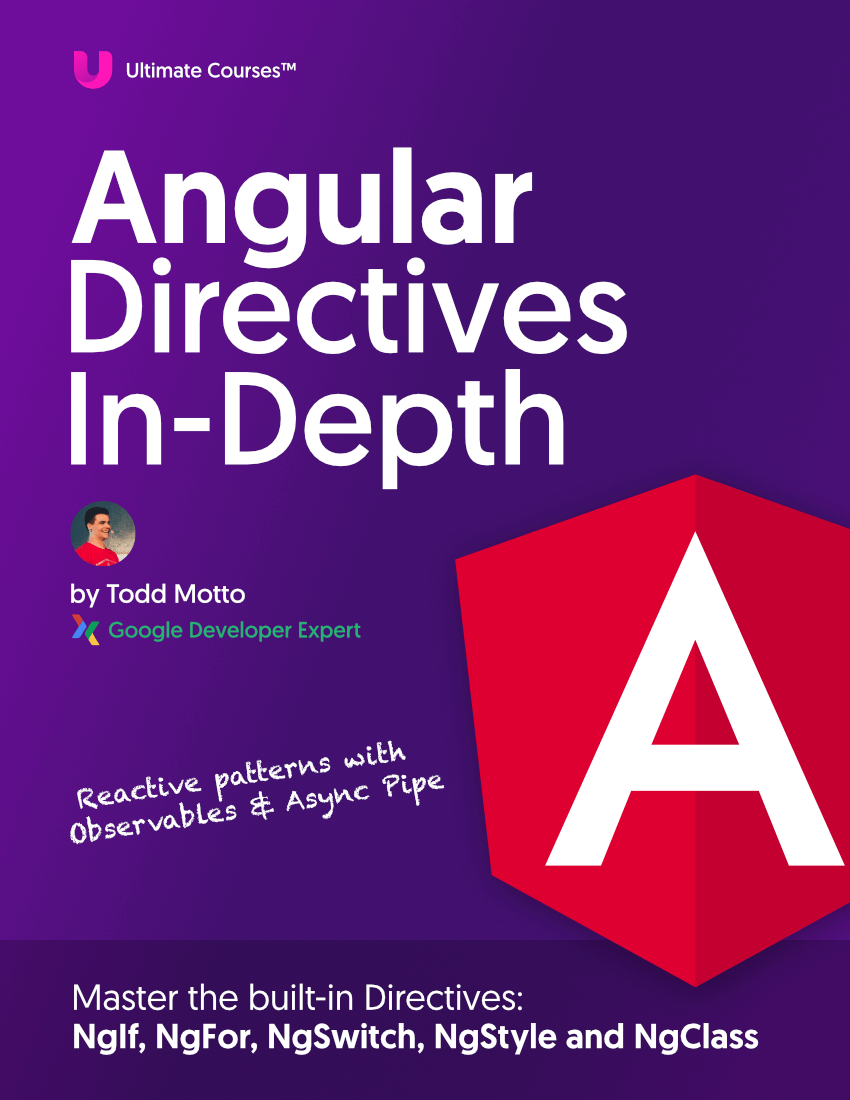
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Let’s sprinkle in the spread operator and log it out with searchParams
:
const Users = () => {
const [searchParams] = useSearchParams();
console.log([...searchParams]); // ▶ [['sort', 'name'], ['order', 'ascending']]
return <div>Users</div>;
};
Well look at that, a multidimensional array (array inside an array). Maybe there’s a method for that to turn it into an object? Of course there is, I’m just building the suspense.
If you’ve not heard of Object.fromEntries
then you’re going to love this:
const Users = () => {
const [searchParams] = useSearchParams();
console.log(Object.fromEntries([...searchParams])); // ▶ { sort: 'name', order: 'asecnding' }
return <div>Users</div>;
};
Well, isn’t that something? Very clean and a nice one-liner to turn our search param query string into a simple object. You’re free to do whatever you like with the object now, go pass it off to the server already or do some filtering.
Monitoring Changes to a Query String with useEffect
Next up, let’s learn how to see changes to a query string with useEffect
. For this, we’re going to pass searchParams
as a dependency into the useEffect
hook, so that the code is executed every time a param changes.
This is great for two things, on the fly filtering and sorting of perhaps a list - but also if you wanted to reuse a component without it having to re-mount every time. It’s all about minimalistic code and those performance gains.
Here’s how to use it with useEffect
, this will run every time the params change AND also when the component mounts the first time, so this could be a good place to read params regardless of how many times you’re referencing them if you’re passing them off to an async function:
const Users = () => {
const [searchParams] = useSearchParams();
useEffect(() => {
const currentParams = Object.fromEntries([...searchParams]);
console.log(currentParams); // get new values onchange
}, [searchParams]);
return <div>Users</div>;
};
Here’s a working example in StackBlitz of what we’ve covered here (I’ve added some query string params to the URL so you can see it in action):
Hopefully this gives you every scenario you might need when getting your query string or reading parameter values from the URL with React Router, which provides us a nice hooks-based wrapper around URLSearchParams
to help us on our way.
💯 Serious about your React skills? Your next step is to take a look at my React courses where you’ll learn React, React Router, Styling and CSS practices, advanced concepts and APIs as well as server-side rendering and much more.
Happy querying!