In this post you’ll learn how to use the new ES6 method Object.is()
in JavaScript.
What is Object.is()?
Introduced in ECMAScript 2015, known as ES2015 or ES6, Object.is() allows us to test equality of JavaScript objects (and primitive values, despite the name).
Object.is()
is very similar to the JavaScript strict comparison operator ===
and can almost be used interchangeably (despite some minor differences which we’ll cover).
We won’t discuss the differences between both ==
and ===
here, as I’ll assume you know what a strict comparison with ===
does. A strict comparison performs no type conversion, known as coercion, compared to non-strict (behind the scenes they both perform type coercion, but strict disregards it when evaluating equality).
In its simplest form, we could use Object.is()
with strings, numbers or objects (and much more):
'abc' === 'abc' // true
Object.is('abc', 'abc') // true
1 === 1 // true
Object.is(1, 1) // true
'1' === 1 // false
Object.is('1', 1) // false
{} === {} // false
Object.is({}, {}) // false
We’ll momentarily explore the differences between Object.is()
and ===
as well, as here they act the same!
When using ===
, we are performing a value-comparison operation.
When using Object.is()
we are performing a check using the SameValue algorithm, which looks like so:
1. If Type(x) is different from Type(y), return false.
2. If Type(x) is Number or BigInt, then
a. Return ! Type(x)::sameValue(x, y).
3. Return ! SameValueNonNumeric(x, y).
🕵️♀️ Check out the official Object.is() ECMAScript standard for a deeper syntax guide!
Really, we’re here to answer one question - why should I use Object.is()
over ===
?
Differences between Object.is() and ===
There are two major differences between Object.is()
and the triple equals comparison ===
. All other operations with Object.is()
and ===
will produce the identical result.
The first difference is that -0
and +0
can now be properly compared:
+0 === -0 // true
Object.is(+0, -0) // false
Really, it comes down to NaN
(Not-a-Number) and how it fixes the behaviour when comparing values that could be NaN
- such as Number('abc')
:
NaN === NaN // false
Object.is(NaN, NaN) // true
Number.NaN === Number.NaN // false
Object.is(Number.NaN, Number.NaN) // true
NaN === Number.NaN // false
Object.is(NaN, Number.NaN) // true
This tells us that Object.is()
was also created to help us to properly distinguish between NaN
values (and even the newly added Number.NaN
).
We also get the added benefit with the Object.is()
prototype method that it follows a more functional programming style.
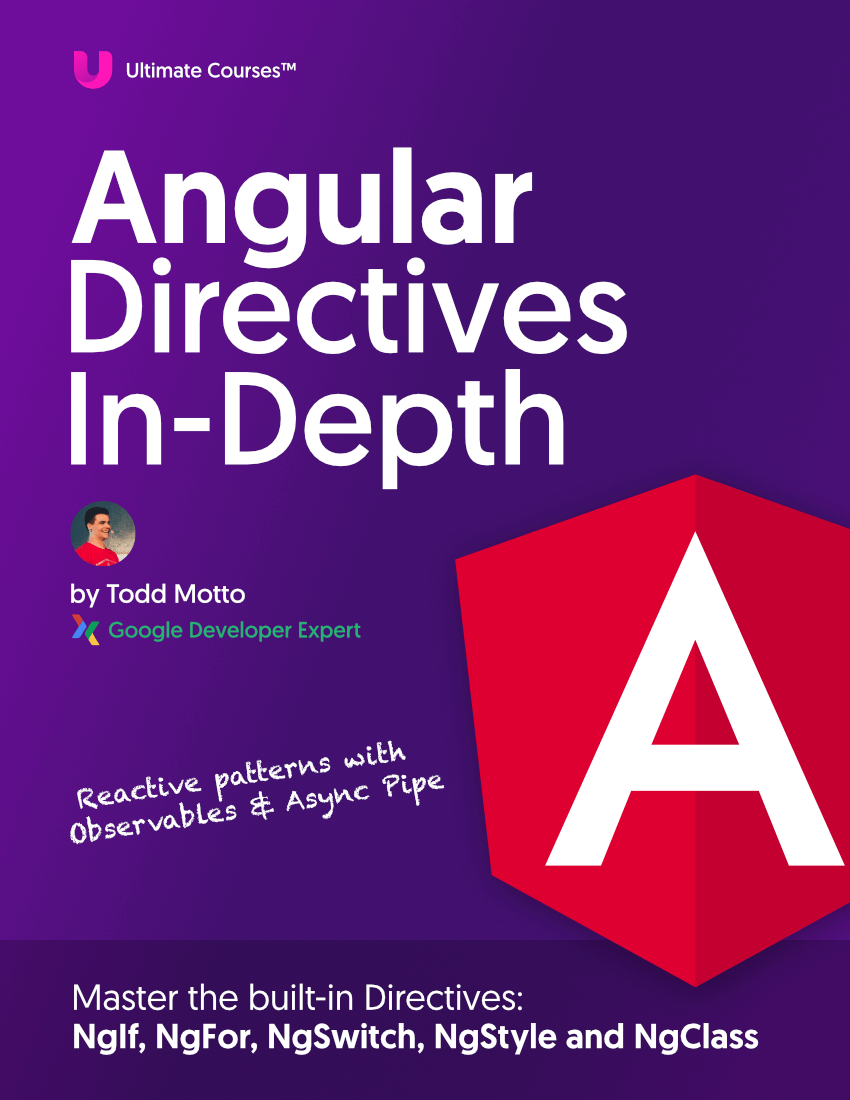
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Other Array prototype methods such as Array ForEach and Array Reduce also slot nicely into a functional programming style. However, I will admit the code is likely cleaner using ===
, so use what makes sense.
Check out the examples I’ve created above in the live StackBlitz demo:
Summary
We’ve covered the new ES6 Object.is()
method and compared it to JavaScript’s strict triple equals statement ===
.
If you are serious about your JavaScript skills, your next step is to take a look at my JavaScript courses, they will teach you the full language, the DOM, the advanced stuff and much more!
Learning the differences between the two, we can now choose to use Object.is()
as a better comparison check for NaN
values, as well as adopting a more functional programming style.
Happy coding!