Follow along with the Exploring JavaScript Array Methods series!
- Exploring Array ForEach (you’re here)
- Exploring Array Map
- Exploring Array Filter
- Exploring Array Reduce
- Exploring Array Some
- Exploring Array Every
- Exploring Array Find
What is Array ForEach?
Array ForEach is a method that exists on the Array.prototype
that was introduced in ECMAScript 5 (ES5) and is supported in all modern browsers.
Array ForEach is the entry-level loop tool that will iterate over your array and pass you each value (and its index). You could render the data to the DOM for example. ForEach, unlike other array methods, does not return any value.
Think of Array ForEach as: “I want to access my array values one-by-one so I can do something with them”
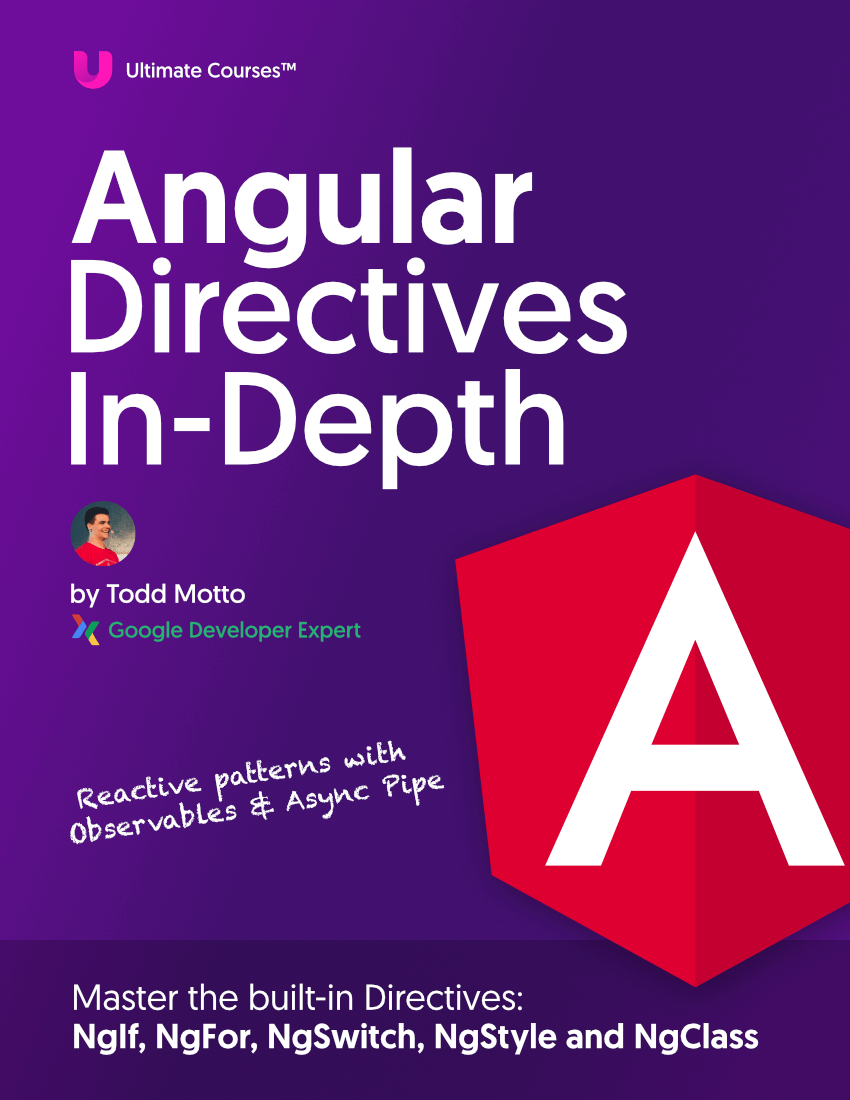
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
You may hear this being called a ForEach loop.
Here’s the syntax for Array ForEach:
array.forEach((value, index, array) => {...}, thisArg);
ForEach is also considered a “modern for…in loop” replacement, despite behaving differently and having less flexibility.
ForEach doesn’t allow us to break
the loop or even loop backwards, which may be a common scenario you’ll run into - so it’s certainly not a flat-out replacement. However, it’s a pretty standard approach nowadays.
Array ForEach syntax deconstructed:
- ForEach’s first argument is a callback function that exposes these parameters:
value
(the current element)index
(the element’s index - fairly commonly used)array
(the array we are looping - rarely used)- Inside the body of the function we would access
value
and do something with us, sum values, inject template into the DOM, anything you like
- Every’s second argument
thisArg
allows the this context to be changed
See the ECMAScript Array ForEach specification!
In its simplest form, here is how ForEach behaves:
['a', 'b', 'c', 'd'].forEach(function(item, index) {
console.log(item, index);
});
We would then see something like this being printed out in the console
(note the index number too):
a 0
b 1
c 2
d 3
Accessing each array element, and its index, couldn’t be easier!
Once your array has been ‘looped’, ForEach will exit and your JavaScript program will continue.
Using Array ForEach
Here’s our data structure that we’ll be using Array ForEach with (we’ll be using this same array throughout this series):
const items = [
{ id: '🍔', name: 'Super Burger', price: 399 },
{ id: '🍟', name: 'Jumbo Fries', price: 199 },
{ id: '🥤', name: 'Big Slurp', price: 299 }
];
Let’s move to a more realistic scenario - we want to display the details of each array object to the user, the id
, name
and price
!
We can construct a new <li>
template for each array element, and inject it into the DOM:
const app = document.querySelector('#app');
items.forEach(item => {
app.innerHTML += `
<li>
${item.id} ${item.name} - ${(item.price / 100).toFixed(2)}
</li>
`;
});
Nicely done. Rendering the data to the DOM is a great use case for ForEach, notice how we also do not return
anything as well (which we will have to with most other array methods in this series).
Give the live Array ForEach demo a try:
Bonus: ForEach-ing without ForEach
Let’s check out a for...in
loop example that mimics the behaviour of Array ForEach:
const app = document.querySelector('#app');
for (let i = 0 ; i < items.length; i++) {
const item = items[i];
app.innerHTML += `
<li>
${item.id} ${item.name} - ${(item.price / 100).toFixed(2)}
</li>`;
}
Both achieve the identical output, it’s merely how we compose the code.
Whilst technically the ForEach method on JavaScript arrays is more “functional” style, it does not return anything - therefore cannot be chained as part of a series of operators that transform data, for example .map().filter()
which we’ll learn about shortly.
ForEach is a nice and clean API and should be thought about as a one-way street. Here, I’m simply using it to render, there is no further action taking place and I get no return value from the method call.
We can also loop backwards and have the flexibility to break
with the for...in
loop.
Using ForEach or the newer for...of
is preferred for simple looping.
Summary
You’ve now learned how to use Array ForEach to loop over your array elements and render some data.
ForEach is a great place to get started with JavaScript loops. Moving from a traditional for...in
loop, the ForEach method can be introduced to bring a more functional approach and style to your programming.
If you’re enjoying this series, please keep following! To learn more JavaScript practices at this level and comprehensively, I’ve put together some online videos that explore the entire JavaScript language and the DOM - I’d encourage you to check them out if you are serious about JavaScript.
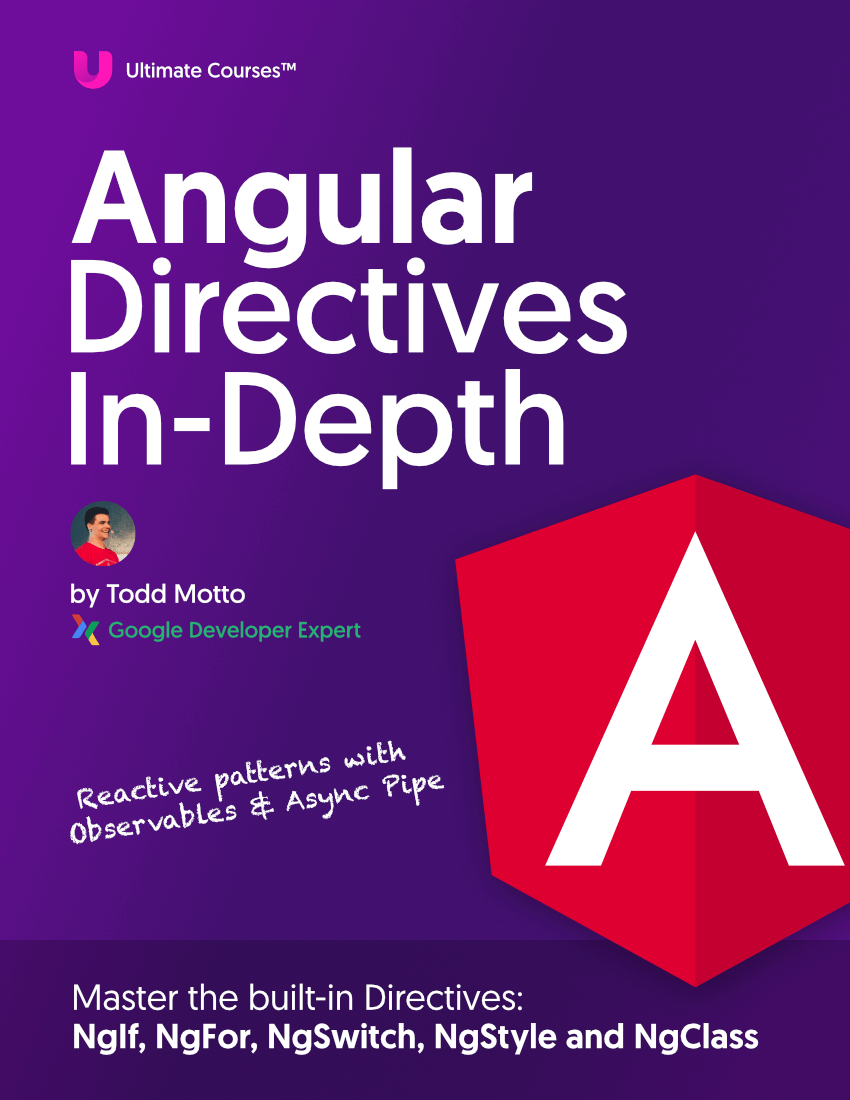
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Further tips and tricks:
- ForEach is great for simple looping as it doesn’t return any values
- You cannot
break
orcontinue
items inside a ForEach - You cannot ForEach in reverse, use
for...in
orfor...of
- You can access the array you’re looping in the third argument of the callback function
- You can change the
this
context via a second argument to.forEach(callback, thisArg)
so that any references tothis
inside your callback function point to your object - You can use arrow functions with ForEach but remember that
this
will be incorrect if you also supply athisArg
due to arrow functions not having athis
context - Using ForEach will skip empty array slots
- You shouldn’t need to in this day and age of evergreen browsers, but use a polyfill for older browsers if necessary
Thanks for reading, happy Foreaching!
Go to the next article in Exploring JavaScript Array Methods - Array Map!