In this post you’ll learn how to use JavaScript to find a substring inside a string.
Let’s assume we have a secret passcode 'bacon'
and want to check if it exists in another string.
Here we’ll use findme
to demonstrate the string we want to check:
const passcode = 'bacon';
const findme = `8sowl0xebaconxjwo98w`;
Visually we can see that findme
contains 'bacon'
but how do we get a yes/no answer in JavaScript?
We can introduce a new feature in ES6, the String.prototype.includes
method, which will return us a Boolean value based on whether the substring was found:
const found = findme.includes(passcode);
// true
console.log(found);
🐛 Check the browser support for String includes! Either compile your code with Babel or provide a polyfill.
This is a really nice method and it gives us a straight-up Boolean answer - but it wasn’t always this way.
First, came String.prototype.indexOf
- where we can ask for the index of the start of the string, should it match. If it does match, we get the index, or we get -1
, so naturally our safety check does exactly this:
const index = findme.indexOf(passcode);
// true
console.log(index !== -1);
The -1
isn’t the best looking code, it feels a bit archaic - so prefer the use of String.prototype.includes
where appropriate.
You could also use the bitwise operator ~
instead as a little shorthand, and we’ll leave it at that:
const index = !!~findme.indexOf(passcode);
// true
console.log(index);
The !!~
converts the resulting bitwise expression to a Boolean. It’s basically a fancy way of forcing indexOf
to return us true
or false
in one line.
Summary
We’ve covered the new ES6 String.prototype.includes
method and compared it to the previously-favoured String.prototype.indexOf
method.
The different between the two is includes
will return you a Boolean and indexOf
will return you a number.
Typically includes
is the go-to method unless you need to know the index!
🕵️♀️ Learn more about the indexOf method for finding a string inside a string
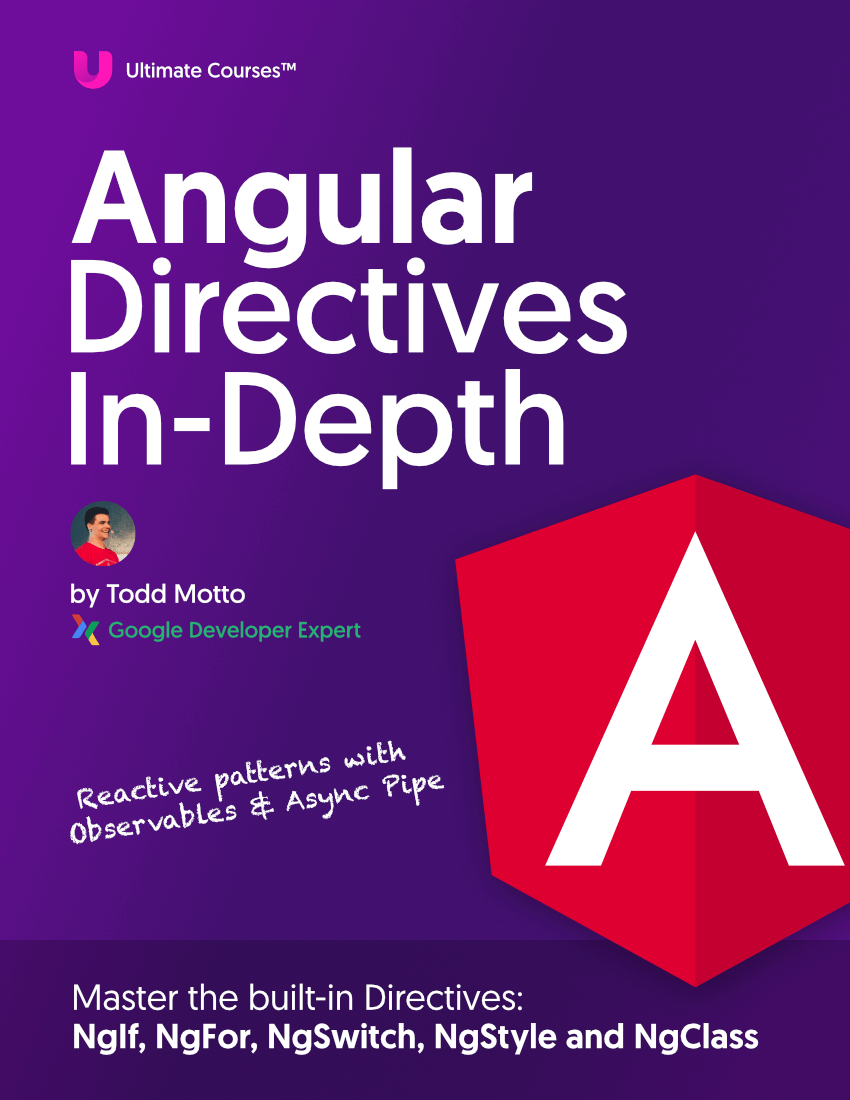
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
I hope you enjoyed the post, and if you’d love to learn more please check out my JavaScript courses, where you’ll learn everything you need to know to be extremely good and proficient at the language, DOM and much more advanced practices. Enjoy and thanks for reading!
Happy coding!