Follow along with the Exploring JavaScript Array Methods series!
- Exploring Array ForEach
- Exploring Array Map
- Exploring Array Filter (you’re here)
- Exploring Array Reduce
- Exploring Array Some
- Exploring Array Every
- Exploring Array Find
What is Array Filter?
Array Filter is a method that exists on the Array.prototype
that was introduced in ECMAScript 5 (ES5) and is supported in all modern browsers.
Array Filter allows us to conditionally return certain elements from our array, into a new array. It’s commonly used to remove items from an array by excluding them from the result.
Think of Array Filter as: “I want a new array containing just the items I need”
With each iteration of a Filter loop, you will need to return an expression that Filter evaluates either true
or false
.
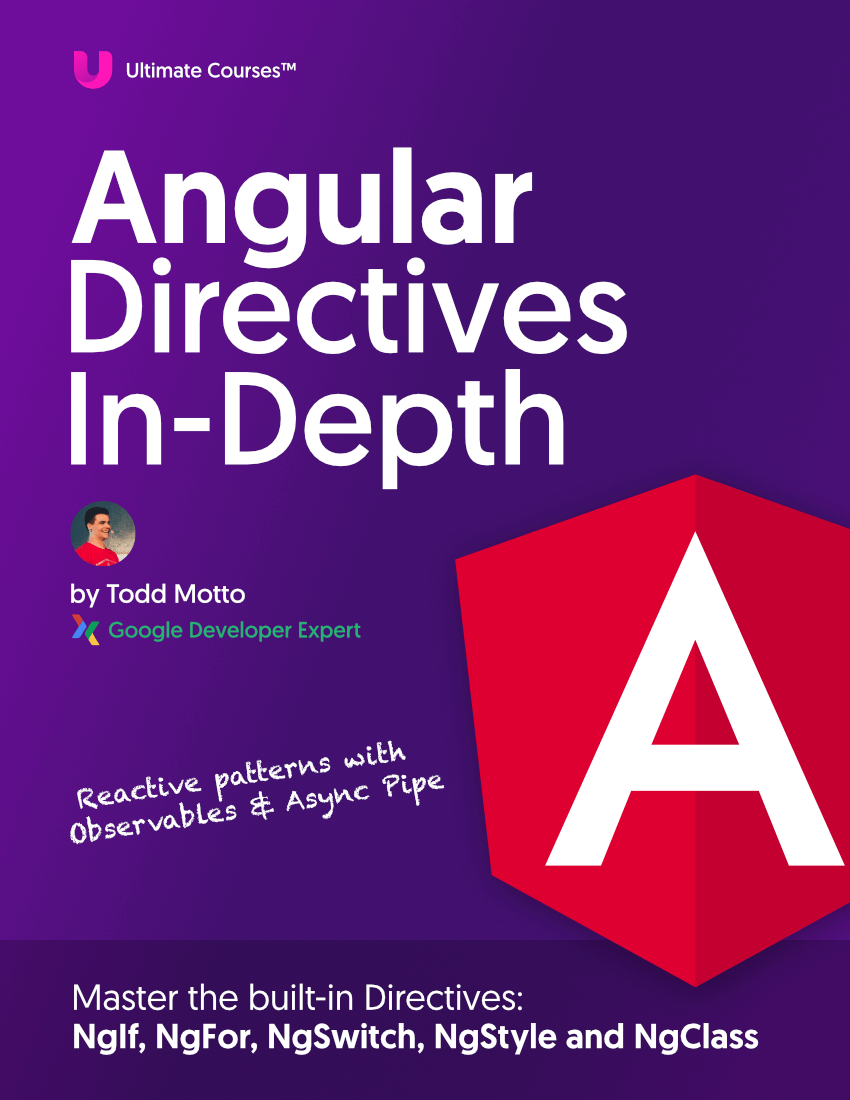
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
The key to understanding Filter is to realise your callback is returning an expression that will evaluate to true
or false
.
Array elements that evaluate to true
are stored in a new array - and items that evaluate to false
are excluded. Once Filter has completed, you can access the new array of your values.
Here’s the syntax for Array Filter:
const returnValue = array.filter((value, index, array) => {...}, thisArg);
Our returnValue
will contain our new array of filtered return values.
Array Filter syntax deconstructed:
- Filter’s first argument is a callback function that exposes these parameters:
value
(the current element)index
(the element’s index - sometimes used with Filter)array
(the array we are looping - rarely used)- Inside the body of the function we need to
return
an expression which will evaluate to a Boolean (true
orfalse
)
- Filter’s second argument
thisArg
allows the this context to be changed
See the ECMAScript Array Filter specification!
In its simplest form, here is how Filter behaves:
const array = [true, true, false];
// [true, true]
console.log(array.filter(Boolean));
Array Filter expects us to return an expression that will evaluate true
or false
, we can go straight to the finish line and make it easier by supplying literally true
and false
array values.
I’m using JavaScript’s Boolean
object to coerce the array element to a Boolean. As our array already contains Boolean values, any false
values are omitted.
Notice how Filter is also returning multiple values, compared to its closely related sibling method Array Find.
Using Array Filter
Here’s our data structure that we’ll be using Array Filter with:
const items = [
{ id: '🍔', name: 'Super Burger', price: 399 },
{ id: '🍟', name: 'Jumbo Fries', price: 199 },
{ id: '🥤', name: 'Big Slurp', price: 299 }
];
Here let’s assume we want to create a new array of more expensive items in this array, we can consider “expensive” to be 199
and above.
Let’s return an expression now that compares each item’s price
property with greater than > 199
, which aims to filter out values that aren’t considered expensive:
const expensiveItems = items
.filter(item => item.price > 199);
This would then give us the two items that are considered “expensive” based on our 199
threshold value:
[
{ id: '🍔', name: 'Super Burger', price: 399 },
{ id: '🥤', name: 'Big Slurp', price: 299 }
]
Interestingly, our original items
array remains unmodified, and we have a new collection to deal with now in our expensiveItems
variable. This practice is called an immutable operation as we don’t mutate the initial array.
Give the live demo a try:
Bonus: Filter-ing without Filter
Let’s check out a for…in loop example that mimics the behaviour of Array Filter:
const expensiveItems = [];
for (let i = 0 ; i < items.length; i++) {
const item = items[i];
if (item.price > 199) {
expensiveItems.push(item);
}
}
First we declare expensiveItems
as an empty array. Inside the loop we use pretty much the same logic, but instead of a return
statement we use the Array.prototype.push
method which adds each item to the new expensiveItems
array.
Once the loop as finished, you’re free to work with your new filtered array.
Summary
You’ve now learned how to use Array Filter to filter your array to a specific set of values.
You can think of Filter of like a functional “if statement”. If my array element meets my criteria - give it to us… Else, we don’t want it.
If you are serious about your JavaScript skills, your next step is to take a look at my JavaScript courses, they will teach you the full language, the DOM, the advanced stuff and much more!
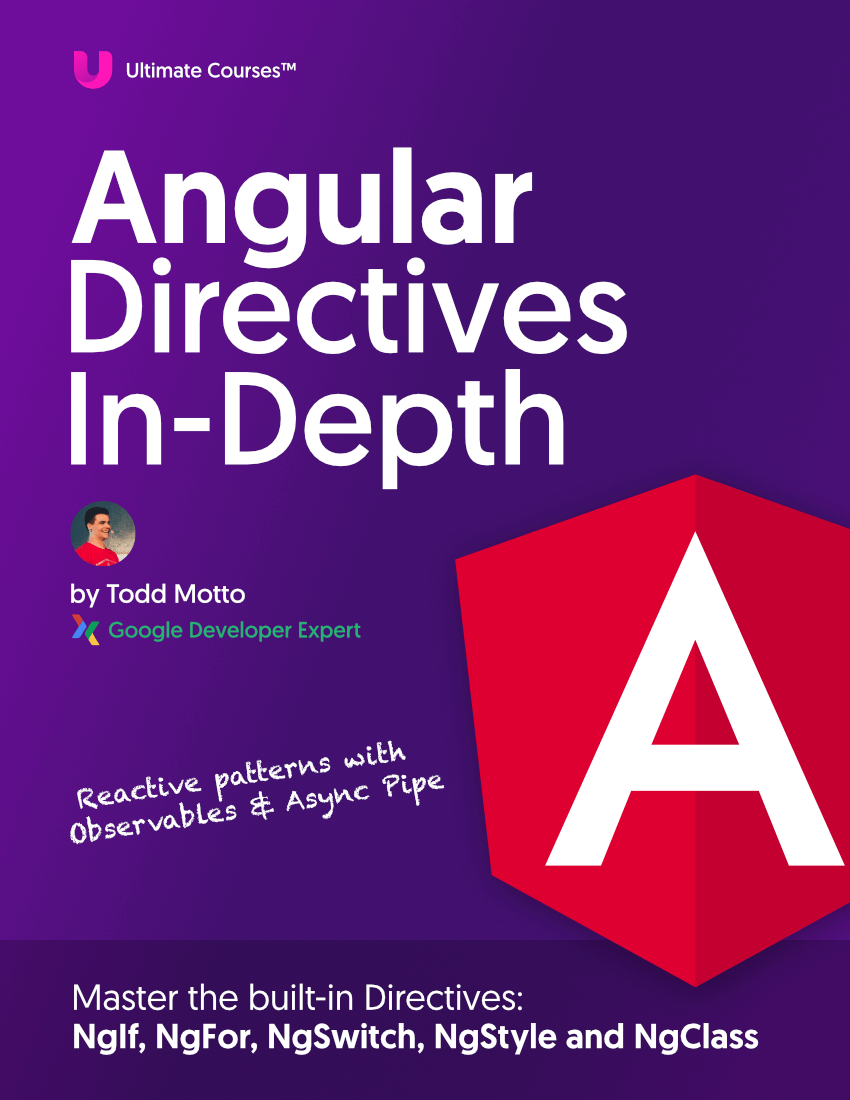
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
Further tips and tricks:
- Filter can be used to return specific values from a source array
- Don’t forget to
return
or your values will beundefined
which coerces tofalse
(so an undetected bug could be introduced!) - The easiest way to get all truthy values in the array is by using
.filter(Boolean)
- Don’t forget to
return
or your values will beundefined
- Filter will shallow copy your object references into the new array
- Filter is also similar to Find, but for multiple items!
- You can access the array you’re looping in the third argument of the callback
- You can change the
this
context via a second argument to.filter(callback, thisArg)
so that any references tothis
inside your callback point to your object - You can use arrow functions with Filter but remember that
this
will be incorrect if you also supply athisArg
due to arrow functions not having athis
context - Using Filter will skip empty array slots
- You shouldn’t need to in this day and age of evergreen browsers, but use a polyfill for older browsers if necessary
Thanks for reading, happy Filtering!
Go to the next article in Exploring JavaScript Array Methods - Array Reduce!