In this post you’ll learn how to handle 404 routes in React Router and provide a fallback component for displaying an imfamous 404 Page Not Found
error to the user.
In React and React Router, we’re in the land of SPA (Single Page Apps), so naturally there will be no “404” page - but a component instead. The component will need displaying if a route isn’t recognised.
Thankfully, this is nice and easy in React Router!
Inside the <Switch />
component from React Router, we can declare a final <Route />
at the bottom of the route declarations.
🎉 React Router’s
<Switch>
component will render the first matched component, making it the perfect tool for us!
This means if the routes above haven’t matched, the only possible solution is we’ve hit a route that doesn’t actually exist.
I’ve setup a Hello
and NotFound
component and added them inside our App
:
import React from 'react';
import { render } from 'react-dom';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Hello from './Hello';
import NotFound from './NotFound';
const App = () => (
<Router>
<Switch>
<Route exact path="/" component={Hello} />
<Route component={NotFound} />
</Switch>
</Router>
);
render(<App />, document.getElementById('root'));
Were you expecting more? That’s it! You don’t need to worry about path
or exact
, we can simply specify the component
to render.
🙌 By not using
path
the route will always match, and it will only match when no other matches above were found.
Let’s use React Router’s <Link to="/oops" />
component inside our Hello
component to let the user click and route to /oops
- a route that does not exist in our app:
import React from 'react';
import { Link } from 'react-router-dom';
const Hello = () => (
<div>
<p>Click to route to "/oops" which isn't a registered route:</p>
<Link to="/oops">Let's go</Link>
</div>
);
export default Hello;
And here’s the NotFound
component, it’s just a regular functional component that uses React Router’s <Link to="/">
to head back to the home page, or anywhere else you’d like in your app:
import React from 'react';
import { Link } from 'react-router-dom';
const NotFound = () => (
<div>
<h1>404 - Not Found!</h1>
<Link to="/">Go Home</Link>
</div>
);
export default NotFound;
Check out the live Stackblitz example below and watch the URL change (feel free to change the URL to anything and it will 404
on you):
Summary
A nice and easy post for React Router and how to provide a fallback component for when a route is not found.
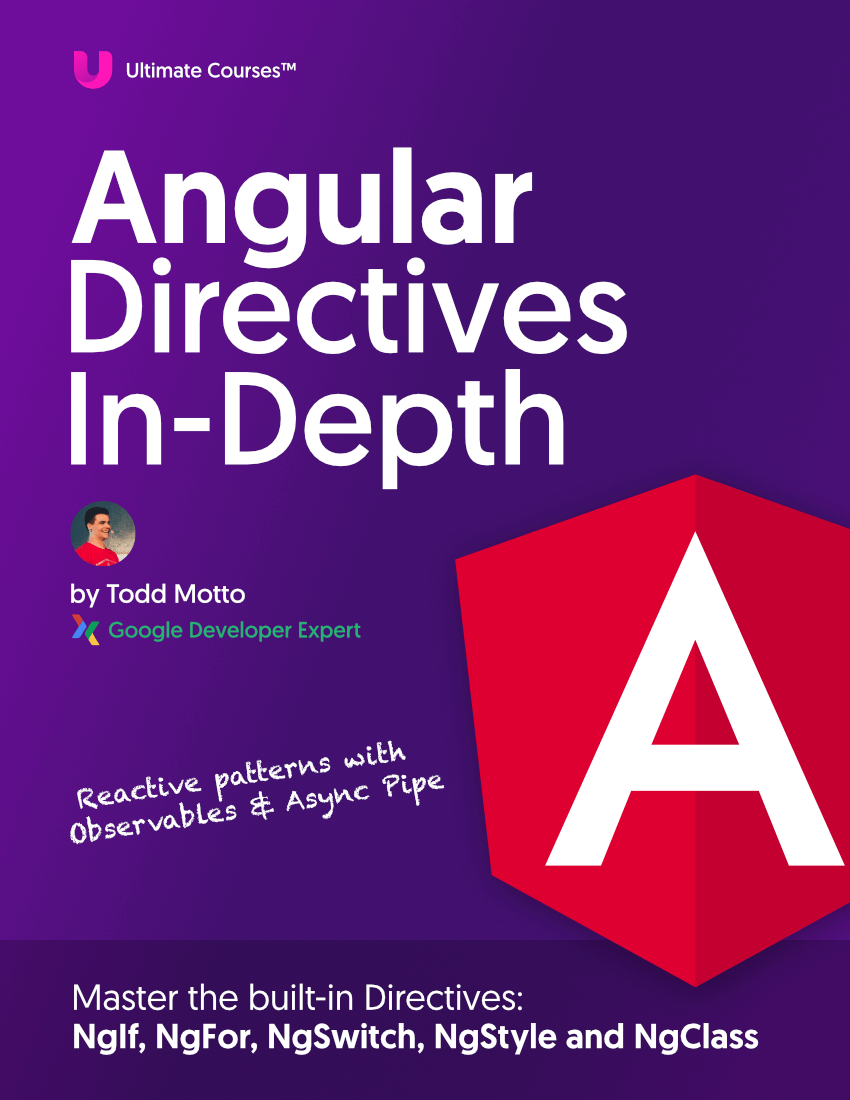
Free eBook
Directives, simple right? Wrong! On the outside they look simple, but even skilled Angular devs haven’t grasped every concept in this eBook.
-
Observables and Async Pipe
-
Identity Checking and Performance
-
Web Components <ng-template> syntax
-
<ng-container> and Observable Composition
-
Advanced Rendering Patterns
-
Setters and Getters for Styles and Class Bindings
I hope you enjoyed the post, and if you’d love to learn more please check out the React courses we’re working on where you’ll learn everything you need to know to be extremely good and proficient at React and the surrounding ecosystem, hooks and beyond!
By using React Router’s <Switch />
and adding the NotFound
component at the bottom of our <Route />
declarations we are able to ensure that the route only works when we hit an unregistered route.
Happy coding!